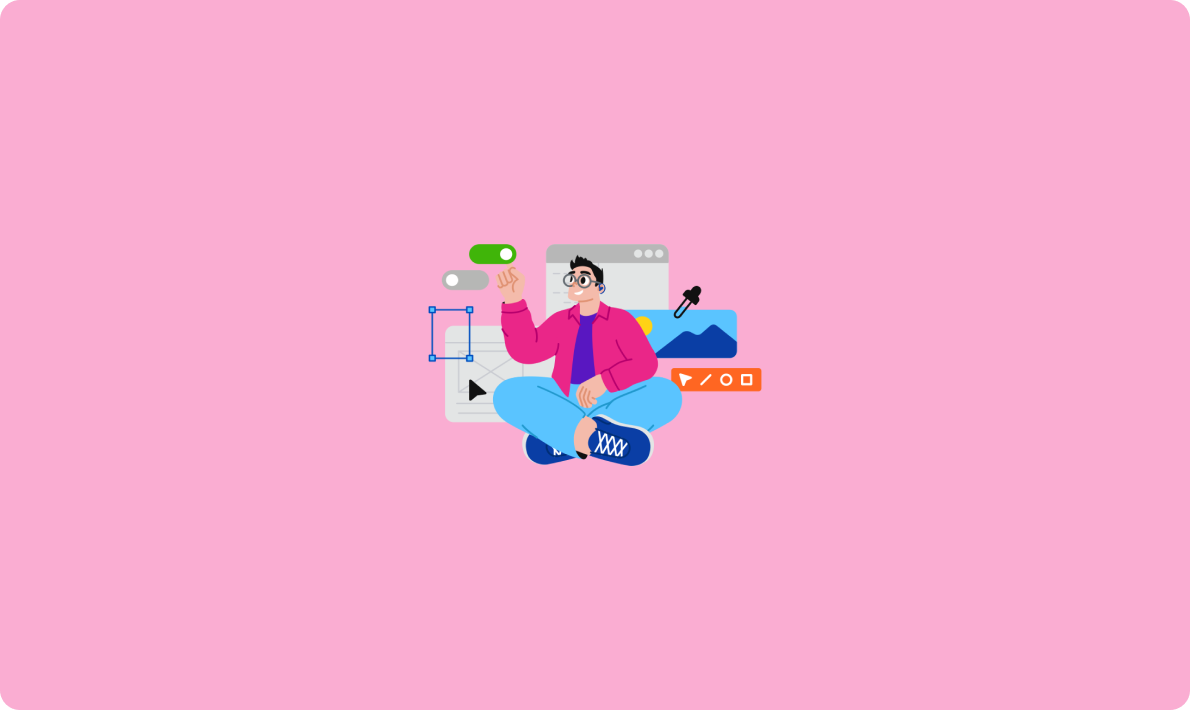
API開發中的日志記錄價值
import json
def create_customer(access_token, customer_data):
url = f"https://api.weibanzhushou.com/v1/customers?access_token={access_token}"
headers = {'Content-Type': 'application/json'}
response = requests.post(url, data=json.dumps(customer_data), headers=headers)
return response.json()
access_token = "your_access_token"
customer_data = {
"name": "張三",
"phone": "13800138000",
"email": "zhangsan@example.com",
"company": "ABC公司"
}
response = create_customer(access_token, customer_data)
print(response)
微伴助手 API 的一個重要功能是消息推送。通過 API,企業可以向指定的用戶或部門發送通知、公告等信息。這在日常工作中非常有用,例如發布公司新聞、提醒員工會議時間等。
以下是 Python 語言的示例代碼,展示如何通過微伴助手 API 發送消息:
import requests
import json
def send_message(access_token, user_id, message):
url = f"https://api.weibanzhushou.com/v1/messages?access_token={access_token}"
payload = {
"user_id": user_id,
"message_type": "text",
"content": message
}
headers = {'Content-Type': 'application/json'}
response = requests.post(url, data=json.dumps(payload), headers=headers)
return response.json()
access_token = "your_access_token"
user_id = "UserID1"
message = "Hello, this is a test message."
response = send_message(access_token, user_id, message)
print(response)
微伴助手提供了豐富的任務管理接口,可以幫助企業管理員輕松地管理任務的創建、更新、刪除等操作。這對于維護企業內部的任務分配和進度跟蹤非常重要。
以下是 Python 語言的示例代碼,展示如何通過微伴助手 API 創建一個新任務:
import requests
import json
def create_task(access_token, task_data):
url = f"https://api.weibanzhushou.com/v1/tasks?access_token={access_token}"
headers = {'Content-Type': 'application/json'}
response = requests.post(url, data=json.dumps(task_data), headers=headers)
return response.json()
access_token = "your_access_token"
task_data = {
"title": "完成項目報告",
"description": "請在本周五之前完成項目報告。",
"due_date": "2025-10-15",
"assignee": "UserID1"
}
response = create_task(access_token, task_data)
print(response)
微伴助手提供了多種數據分析接口,幫助企業管理者做出更好的決策。例如,獲取銷售數據、客戶反饋等。
以下是 Python 語言的示例代碼,展示如何通過微伴助手 API 獲取銷售數據:
import requests
def get_sales_data(access_token, start_date, end_date):
url = f"https://api.weibanzhushou.com/v1/reports/sales?access_token={access_token}&start_date={start_date}&end_date={end_date}"
response = requests.get(url)
return response.json()
access_token = "your_access_token"
start_date = "2025-09-01"
end_date = "2025-09-30"
sales_data = get_sales_data(access_token, start_date, end_date)
print(sales_data)
下表展示了不同類型 API 請求的日均調用量及主要功能對比:
API類型 | 日均調用量(萬次) | 主要功能 |
客戶管理 | 50 | 創建、更新、查詢客戶 |
消息推送 | 30 | 向指定用戶或部門發送通知 |
任務管理 | 40 | 創建、更新和刪除任務 |
數據分析 | 60 | 提供各種統計分析接口,幫助管理決策 |
微伴助手 API 調試工具(如 Apifox)可以幫助開發者快速測試和調試 API。通過這些工具,開發者可以模擬請求并查看響應結果,從而更快地發現問題并進行修復。
以下是使用 Apifox 測試微伴助手 API 的示例代碼:
# 在 Apifox 中配置請求
POST /v1/customers?access_token=your_access_token
Content-Type: application/json
{
"name": "李四",
"phone": "13800138001",
"email": "lisi@example.com",
"company": "XYZ公司"
}
微伴助手 API 的安全性是其核心優勢之一。為了確保數據傳輸的安全性,所有請求都必須經過簽名驗證。簽名算法通常基于 HMAC-SHA256,開發者需要在請求中包含簽名信息。
以下是 Python 語言的示例代碼,展示如何生成簽名:
import hashlib
import hmac
import time
def generate_signature(token, timestamp, nonce):
tmp_arr = [token, str(timestamp), nonce]
tmp_arr.sort()
tmp_str = ''.join(tmp_arr)
signature = hmac.new('your_secret'.encode('utf-8'), tmp_str.encode('utf-8'), hashlib.sha256).hexdigest()
return signature
token = "your_token"
timestamp = int(time.time())
nonce = "nonce"
signature = generate_signature(token, timestamp, nonce)
print(signature)
為了提高 API 的性能,可以采取以下幾種措施:
以下是使用緩存的示例代碼:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
def get_cached_customers():
if r.exists('customers'):
return r.get('customers')
else:
customers = fetch_customers_from_db() # 假設這是一個從數據庫獲取客戶數據的函數
r.set('customers', json.dumps(customers))
return json.dumps(customers)
customers = get_cached_customers()
print(customers)
微伴助手 API 作為一個全面且靈活的開放平臺,不僅為企業和個人開發者提供了豐富的接口選擇,還通過清晰的文檔和技術支持,幫助他們快速成長和發展。無論是想要提升工作效率、優化內部管理還是增強用戶體驗,微伴助手 API 都能提供有效的解決方案。通過本文的介紹,希望能為讀者帶來對微伴助手 API 更深入的理解,并激發更多創新的應用場景。