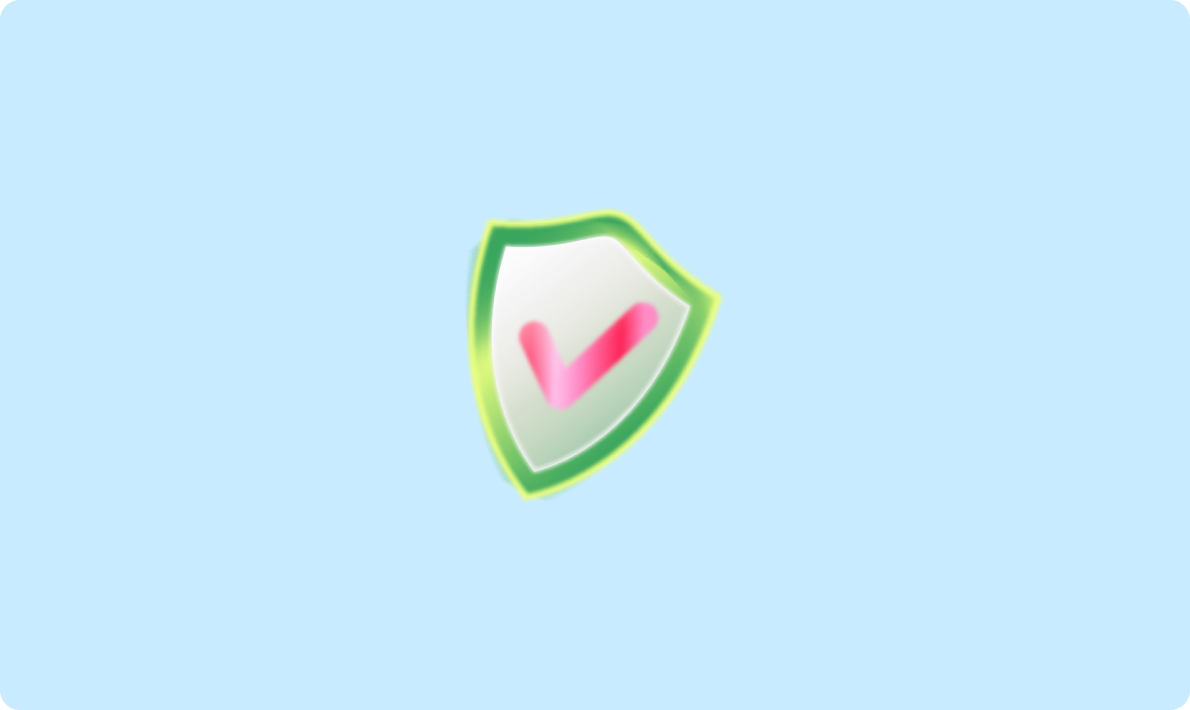
一文講透 AI Agent 與 AI Workflow 的區(qū)別和深度解析:從自動(dòng)化到智能化的演進(jìn)
滑動(dòng)驗(yàn)證碼:需要模擬滑動(dòng)的操作,如 Geetest
。
點(diǎn)擊驗(yàn)證碼:需要用戶點(diǎn)擊特定的圖像區(qū)域,如交通標(biāo)志、汽車等。
行為驗(yàn)證碼:通過監(jiān)測用戶行為(如鼠標(biāo)軌跡)來判定是否為人類。
特殊復(fù)雜驗(yàn)證:如語音、郵箱、手機(jī)短信、二維碼掃描等
常見的破解策略有:
2Captcha
、AntiCaptcha
等,通過自動(dòng)化服務(wù)來識別驗(yàn)證碼。Playwright
的鼠標(biāo)和鍵盤 API
來模擬用戶行為。cookies
)來跳過驗(yàn)證碼。對于一些頻繁遇到驗(yàn)證碼的頁面,手動(dòng)輸入驗(yàn)證碼雖然簡便,但無法完全自動(dòng)化。適用于少量數(shù)據(jù)抓取場景。
當(dāng)遇到驗(yàn)證碼時(shí),手動(dòng)輸入后繼續(xù)抓取。此方法簡單直接,但顯然無法適應(yīng)大規(guī)模爬取。在 Playwright
中,可以使用
page.pause()
方法暫停腳本執(zhí)行,等待手動(dòng)輸入驗(yàn)證碼。
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch({ headless: false });
const page = await browser.newPage();
await page.goto('https://www.example.com/login');
// 輸入用戶名和密碼
await page.fill('#username', 'your-username');
await page.fill('#password', 'your-password');
// 暫停腳本,等待手動(dòng)輸入驗(yàn)證碼
console.log('請?jiān)跒g覽器中手動(dòng)輸入驗(yàn)證碼,然后按回車?yán)^續(xù)...');
await page.pause();
// 點(diǎn)擊登錄按鈕
await page.click('#login-button');
await browser.close();
})();
使用第三方驗(yàn)證碼識別服務(wù)(如 2Captcha
或 Anti-Captcha
)來自動(dòng)識別驗(yàn)證碼。
reCAPTCHA
或其他圖形驗(yàn)證碼。API
密鑰(如 2Captcha
)。2Captcha
識別驗(yàn)證碼const { chromium } = require('playwright');
const axios = require('axios');
const fs = require('fs');
const path = require('path');
(async () => {
const browser = await chromium.launch({ headless: false });
const page = await browser.newPage();
// 打開包含驗(yàn)證碼的頁面
await page.goto('https://example.com/captcha');
// 截取驗(yàn)證碼圖片
const captchaImg = await page.locator('.captcha-image').screenshot();
fs.writeFileSync(path.join(__dirname, 'captcha.png'), captchaImg);
// 使用 2Captcha API 進(jìn)行驗(yàn)證碼識別
const captchaResult = await solveCaptchaWith2Captcha('YOUR_API_KEY', 'captcha.png');
// 輸入驗(yàn)證碼
await page.fill('#captcha-input', captchaResult);
await page.click('#submit-button');
console.log('驗(yàn)證碼已解決并提交');
await browser.close();
})();
async function solveCaptchaWith2Captcha(apiKey, imagePath) {
const image = fs.readFileSync(path.join(__dirname, imagePath));
const response = await axios.post(http://2captcha.com/in.php?key=${apiKey}&method=userrecaptcha&body=${image}
, image);
const captchaId = response.data.request;
// 查詢識別結(jié)果
const result = await axios.get(http://2captcha.com/res.php?key=${apiKey}&action=get&id=${captchaId}
);
return result.data.request;
}
有些驗(yàn)證碼(如 Geetest
、reCAPTCHA
)通過模擬用戶行為來驗(yàn)證是否為人類。這些驗(yàn)證碼類型要求用戶執(zhí)行某些操作(如滑動(dòng)條、點(diǎn)擊指定區(qū)域等),我們可以使用 Playwright
來模擬這些用戶行為,繞過驗(yàn)證碼。
Playwright
的 mouse API
模擬滑動(dòng)或點(diǎn)擊操作。mouse.move()
、
mouse.down()
、
mouse.up()
等函數(shù)模擬用戶的滑動(dòng)或點(diǎn)擊行為。
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch({ headless: false });
const page = await browser.newPage();
// 打開包含滑動(dòng)驗(yàn)證碼的頁面
await page.goto('https://www.geetest.com/demo');
// 等待驗(yàn)證碼加載
await page.waitForSelector('.geetest_canvas_bg');
// 獲取滑動(dòng)驗(yàn)證碼元素
const slideElement = await page.locator('.geetest_slider_button');
// 模擬滑動(dòng)動(dòng)作
const box = await slideElement.boundingBox();
const startX = box.x + box.width / 2;
const startY = box.y + box.height / 2;
await page.mouse.move(startX, startY);
await page.mouse.down();
await page.mouse.move(startX + 200, startY, { steps: 20 });
await page.mouse.up();
console.log('滑動(dòng)驗(yàn)證碼已解決');
await browser.close();
})();
在一些網(wǎng)站中,驗(yàn)證碼通常只出現(xiàn)在登錄或注冊時(shí)。為了避免每次都遇到驗(yàn)證碼,我們可以通過保存并復(fù)用瀏覽器的登錄狀態(tài)來繞過驗(yàn)證碼。
使用 Playwright
的 storageState
功能保存登錄狀態(tài),并在后續(xù)腳本中復(fù)用。
(Cookies)
。Cookies
,跳過驗(yàn)證碼。const { chromium } = require('playwright');
// 保存登錄狀態(tài)
(async () => {
const browser = await chromium.launch({ headless: false });
const context = await browser.newContext();
const page = await context.newPage();
await page.goto('https://www.example.com/login');
await page.fill('#username', 'your-username');
await page.fill('#password', 'your-password');
await page.click('#login-button');
// 保存登錄狀態(tài)
await context.storageState({ path: 'login_state.json' });
await browser.close();
})();
// 復(fù)用登錄狀態(tài)
(async () => {
const browser = await chromium.launch({ headless: false });
const context = await browser.newContext({ storageState: 'login_state.json' });
const page = await context.newPage();
await page.goto('https://www.example.com');
console.log(await page.content());
await browser.close();
})();
playwright.chromium.connect_over_cdp()
連接到已打開的 Chromium
瀏覽器實(shí)例,復(fù)用登錄狀態(tài),可以避免每次啟動(dòng)瀏覽器時(shí)都要重新登錄或解決驗(yàn)證碼。
const { chromium } = require('playwright');
(async () => {
// 連接到已打開的 Chromium 瀏覽器實(shí)例
const browser = await chromium.connectOverCDP('http://localhost:9222');
const context = browser.contexts()[0];
const page = context.pages()[0];
await page.goto('https://www.example.com');
console.log(await page.content());
await browser.close();
})();
在爬蟲開發(fā)中,驗(yàn)證碼是一個(gè)常見的反爬蟲手段。面對驗(yàn)證碼,我們可以通過以下策略應(yīng)對:
Playwright
模擬用戶行為(滑動(dòng)、點(diǎn)擊等),繞過驗(yàn)證碼。對于更深入的技術(shù),如何復(fù)用登錄信息以及使用
connect_over_cdp()
方法,我們將在后續(xù)的專題文章中進(jìn)一步展開討論。如果你覺得本系列教程對你有幫助,還請 點(diǎn)個(gè)贊,關(guān)個(gè)注,下次更新不迷路!
原文轉(zhuǎn)載自:https://mp.weixin.qq.com/s/g-AdKRA-Sn9nqbI8jUiohA
一文講透 AI Agent 與 AI Workflow 的區(qū)別和深度解析:從自動(dòng)化到智能化的演進(jìn)
實(shí)測告訴你:DeepSeek-R1 7B、32B、671B差距有多大
6個(gè)大模型的核心技術(shù)介紹
太強(qiáng)了!各個(gè)行業(yè)的AI大模型!金融、教育、醫(yī)療、法律
在Sealos 平臺的幫助下一個(gè)人維護(hù)著 6000 個(gè)數(shù)據(jù)庫
通義萬相,開源!
使用Cursor 和 Devbox 一鍵搞定開發(fā)環(huán)境
DeepSeekMath:挑戰(zhàn)大語言模型的數(shù)學(xué)推理極限
新型脈沖神經(jīng)網(wǎng)絡(luò)+大模型研究進(jìn)展!