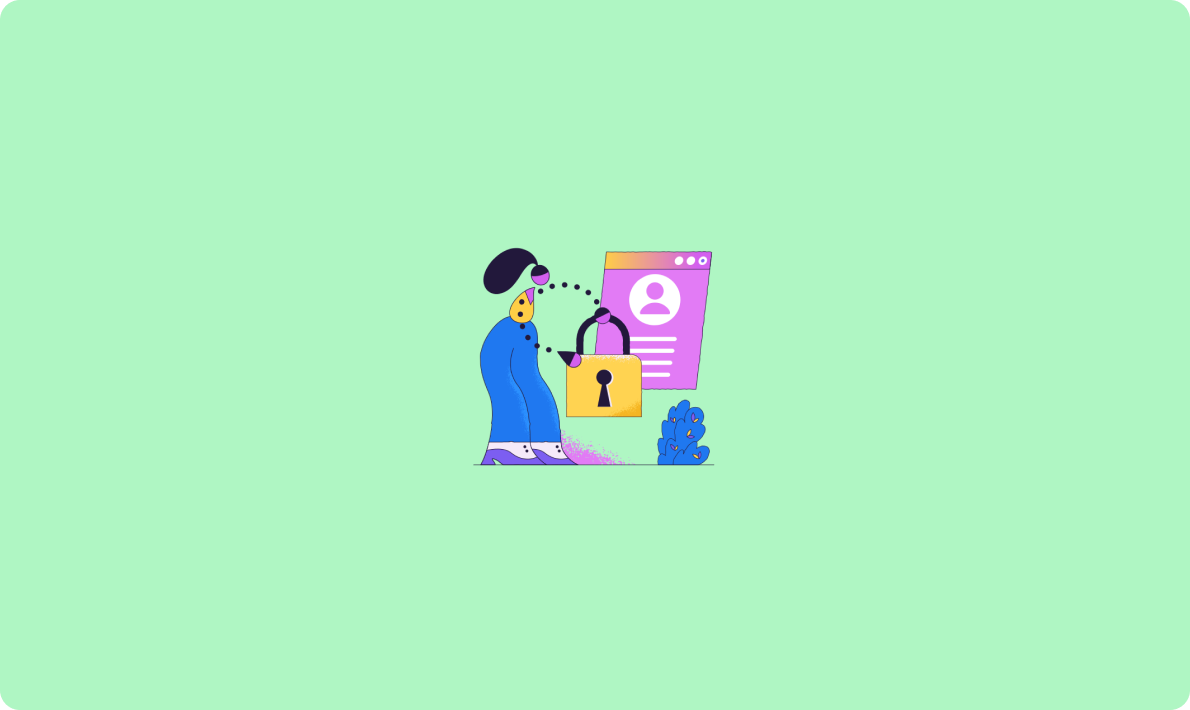
理解 Composition API Vue 的基礎與模式
from langchain_community.chat_models.tongyi import ChatTongyi
# 設置 API 密鑰 (替換為自己的API_Key)
os.environ["DASHSCOPE_API_KEY"] = 'YOUR_SECRET_KEY'
# 初始化 ChatTongyi 模型
llm = ChatTongyi()
# 給出問題
response = llm.invoke('你是誰?')
#獲取回復
print(response.content)
那我們要講的第一個鏈就是最基礎的——LLM Chain。這個鏈其實在使用上和我們第一節(jié)課聊到的非常類似,只是在具體的用法上更加靈活。比如我們能夠直接使用LLM Chain就能把記憶模塊以及結構化輸出等模塊直接鏈接在里面,下面我們就來一起學習一下這個模塊吧!
LLMChain 是 LangChain 提供的一個用于構建邏輯鏈的模塊,它結合了大語言模型(LLM)和提示模板(Prompt Template),從而實現(xiàn)對模型交互邏輯的封裝。通過 LLMChain,開發(fā)者可以:
簡單來說,LLMChain 是開發(fā)者與 LLM 交互的橋梁。
LLMChain 的核心組件:
在使用 LLMChain 時,需要理解以下三個核心組件:
以下是一個完整的代碼示例,展示如何使用 LLMChain 與 ChatTongyi 進行簡單的對話:
import os
from langchain_community.chat_models.tongyi import ChatTongyi
from langchain.chains import LLMChain
from langchain.prompts import ChatPromptTemplate
# 設置 API 密鑰
os.environ["DASHSCOPE_API_KEY"] = 'YOUR_SECRET_KEY'
# 初始化 ChatTongyi 模型
llm = ChatTongyi()
# 使用 ChatPromptTemplate 創(chuàng)建提示模板
prompt = ChatPromptTemplate.from_template("{question}")
# 構建對話鏈
conversation = LLMChain(
llm=llm,
prompt=prompt
)
# 輸入對話內容
input_question = "你是誰?"
# 進行對話,使用 invoke 方法傳遞參數(shù)
response_1 = conversation.invoke(input_question)
print(response_1["text"]) # 打印模型的回答
首先,我們需要導入必要的模塊并通過環(huán)境變量設置 API 密鑰,這一步是為了與 ChatTongyi 模型建立連接。通過?os.environ["DASHSCOPE_API_KEY"]
,我們將密鑰信息傳遞給系統(tǒng),確保模型可以正常運行。
import os
from langchain_community.chat_models.tongyi import ChatTongyi
from langchain.chains import LLMChain
from langchain.prompts import ChatPromptTemplate
# 設置 API 密鑰
os.environ["DASHSCOPE_API_KEY"] = 'YOUR_SECRET_KEY'
接下來是初始化模型。在代碼中,ChatTongyi()
?是一個用于創(chuàng)建大語言模型實例的方法。它負責加載并準備模型以供后續(xù)使用。
# 初始化 ChatTongyi 模型
llm = ChatTongyi()
然后,我們通過?ChatPromptTemplate.from_template("{question}")
?創(chuàng)建了一個提示模板。在模板中,{question}
?是一個占位符,用于動態(tài)填充用戶輸入的問題。這種模板化的設計,讓與模型的交互變得更加靈活。
# 使用 ChatPromptTemplate 創(chuàng)建提示模板
prompt = ChatPromptTemplate.from_template("{question}")
接下來的核心步驟是構建對話鏈。通過?LLMChain
,我們將大語言模型和提示模板結合在一起,形成一個可用的交互鏈條。這個鏈條封裝了底層邏輯,開發(fā)者只需要專注于輸入和輸出。
# 構建對話鏈
conversation = LLMChain(
llm=llm,
prompt=prompt
)
最后,通過?invoke
?方法,我們向對話鏈傳遞了用戶的問題,即?input_question
,模型根據(jù)提示模板生成回答。通過?print(response["text"])
,我們可以直觀地看到模型的輸出結果。
# 輸入對話內容
input_question = "你是誰?"
# 進行對話,使用 invoke 方法傳遞參數(shù)
response_1 = conversation.invoke(input_question)
print(response_1["text"]) # 打印模型的回答
調用完成后,我們就可以在終端里看到大模型給予我們的回復。
我是來自阿里云的大規(guī)模語言模型,我叫通義千問。
但是假如單純只是這樣將大模型和提示詞結合起來并不能展示出langchian的價值,這是因為我們在通義千問官方平臺的調用其實也已經(jīng)能夠做到這一點了,因此其實我們還可以往這個鏈里面加多一些特別的東西,比如說上節(jié)課學習的記憶模塊!
為了將記憶模塊引入,我們需要對代碼進行一些調整。首先,提示詞模板需要新增一個變量?{memory}
?來承接記憶信息。示例如下:
# 創(chuàng)建對話模板,包含歷史對話和當前問題
prompt = ChatPromptTemplate.from_template('''
過往對話:
{history}
用戶的問題是:
{question}
''')
接下來,我們不需要在?.invoke()
?方法中額外手動添加新變量,只需在創(chuàng)建?LLMChain
?時,將?memory
?設置為合適的記憶模塊即可:
# 構建對話鏈,包含模型、提示模板和對話記憶
conversation = LLMChain(
llm=llm,
prompt=prompt,
memory=ConversationBufferMemory(), # 存儲上下文的對話記憶
verbose = True
)
那這個時候,langchian就會自然而然的把這個記憶部分的內容加入進去了!大家可以通過下面的案例進行嘗試:
import os
from langchain_community.chat_models.tongyi import ChatTongyi
from langchain.chains import LLMChain
from langchain.prompts import ChatPromptTemplate
from langchain.memory import ConversationBufferMemory
# 設置 API 密鑰
os.environ["DASHSCOPE_API_KEY"] = 'YOUR_SECRET_KEY'
# 初始化通義千問模型
llm = ChatTongyi()
# 創(chuàng)建對話模板,包含歷史對話和當前問題
prompt = ChatPromptTemplate.from_template('''
過往對話:
{history}
用戶的問題是:
{question}
''')
# 構建對話鏈,包含模型、提示模板和對話記憶
conversation = LLMChain(
llm=llm,
prompt=prompt,
memory=ConversationBufferMemory(), # 存儲上下文的對話記憶
verbose = True
)
# 第一次對話:用戶告訴模型自己的名字
input_question1 = "我是李劍鋒"
response_1 = conversation.invoke(input_question1)
print("模型回答:", response_1["text"])
# 第二次對話:用戶詢問模型的身份
input_question2 = "你是誰?"
response_2 = conversation.invoke(input_question2)
print("模型回答:", response_2["text"])
# 第三次對話:用戶測試模型是否記住了自己的名字
input_question3 = "你還記得我叫什么嗎?"
response_3 = conversation.invoke(input_question3)
print("模型回答:", response_3["text"])
我們從結果中可以得知,模型記住了我的名字!假如我們不希望打印中間的過程內容,我們可以把前面的verbose=False即可。
> Entering new LLMChain chain...
Prompt after formatting:
Human:
過往對話:
用戶的問題是:
我是李劍鋒
> Finished chain.
模型回答: 你好,李劍鋒!很高興認識你。你可以問我任何問題,我會盡力回答它們。或者,如果你需要幫助,我也很樂意提供幫助。
> Entering new LLMChain chain...
Prompt after formatting:
Human:
過往對話:
Human: 我是李劍鋒
AI: 你好,李劍鋒!很高興認識你。你可以問我任何問題,我會盡力回答它們。或者,如果你需要幫助,我也很樂意提供幫助。
用戶的問題是:
你是誰?
> Finished chain.
模型回答: 我是來自阿里云的大規(guī)模語言模型,我叫通義千問。
> Entering new LLMChain chain...
Prompt after formatting:
Human:
過往對話:
Human: 我是李劍鋒
AI: 你好,李劍鋒!很高興認識你。你可以問我任何問題,我會盡力回答它們。或者,如果你需要幫助,我也很樂意提供幫助。
Human: 你是誰?
AI: 我是來自阿里云的大規(guī)模語言模型,我叫通義千問。
用戶的問題是:
你還記得我叫什么嗎?
> Finished chain.
模型回答: 當然記得,你是李劍鋒。
那除了傳入單一問題變量?{current_question}
?外,我們還可以嘗試加入其他變量。例如,如果希望大模型根據(jù)指定的語氣?{tone}
?回復,我們需要對提示詞模板進行調整:
# 創(chuàng)建對話模板,包含多個變量(如歷史對話、當前問題和語氣)
prompt = ChatPromptTemplate.from_template('''
過往對話:
{history}
當前問題:
{current_question}
語氣:
{tone}
''')
另外在調用?.invoke()
?方法時,我們需要以字典的格式傳入多個變量:
# 第一次對話:用戶告訴模型自己的名字,語氣為友好
input_question1 = "我是李劍鋒"
input_tone1 = "友好"
response_1 = conversation.invoke({"current_question": input_question1, "tone": input_tone1})
print("模型回答:", response_1["text"])
# 第二次對話:用戶詢問模型的身份,語氣為正式
input_question2 = "你是誰?"
input_tone2 = "正式"
response_2 = conversation.invoke({"current_question": input_question2, "tone": input_tone2})
print("模型回答:", response_2["text"])
# 第三次對話:用戶測試模型是否記得自己的名字,語氣為隨意
input_question3 = "你還記得我叫什么嗎?"
input_tone3 = "隨意"
response_3 = conversation.invoke({"current_question": input_question3, "tone": input_tone3})
print("模型回答:", response_3["text"])
但是我們會發(fā)現(xiàn)程序無法正常運行,出現(xiàn)的報錯信息為:
ValueError: One input key expected got ['tone', 'current_question']
報錯的核心原因是 ConversationBufferMemory
僅支持單一的輸入鍵,而代碼中傳遞了多個輸入鍵(tone
和 current_question
),導致沖突。通過觀察前面 ConversationBufferMemory
的工作方式可以發(fā)現(xiàn),它只會將提示詞中最關鍵的問題部分(即 current_question
)保存為對話記憶,而不會處理其他額外的變量(如 tone
)。
為了解決這個問題,可以采用以下兩種方法:
tone
和 current_question
合并為一個鍵值后再傳入,避免多個輸入鍵的沖突。ConversationBufferMemory
中指定 input_key
參數(shù),讓其只保存 current_question
,忽略語氣變量的影響。以下是調整后的實現(xiàn)代碼,采用了第二種方式:
# 構建對話鏈,包含模型、提示模板和對話記憶
conversation = LLMChain(
llm=llm,
prompt=prompt,
memory = ConversationBufferMemory(input_key="current_question"),
verbose=True
)
這樣調整了以后我們就會發(fā)現(xiàn)程序能夠正常運行了并給出合適的回復。
> Entering new LLMChain chain...
Prompt after formatting:
Human:
過往對話:
當前問題:
我是李劍鋒
語氣:
友好
> Finished chain.
模型回答: 你好,李劍鋒!很高興認識你。有什么可以幫到你的嗎?或者你只是想聊聊天?
> Entering new LLMChain chain...
Prompt after formatting:
Human:
過往對話:
Human: 我是李劍鋒
AI: 你好,李劍鋒!很高興認識你。有什么可以幫到你的嗎?或者你只是想聊聊天?
當前問題:
你是誰?
語氣:
正式
> Finished chain.
模型回答: 我是來自阿里云的大規(guī)模語言模型,我叫通義千問。
> Entering new LLMChain chain...
Prompt after formatting:
Human:
過往對話:
Human: 我是李劍鋒
AI: 你好,李劍鋒!很高興認識你。有什么可以幫到你的嗎?或者你只是想聊聊天?
Human: 你是誰?
AI: 我是來自阿里云的大規(guī)模語言模型,我叫通義千問。
當前問題:
你還記得我叫什么嗎?
語氣:
隨意
> Finished chain.
模型回答: 當然記得,你好,李劍鋒!有什么事情我可以幫助你嗎?
完整的代碼如下所示:
import os
from langchain_community.chat_models.tongyi import ChatTongyi
from langchain.chains import LLMChain
from langchain.prompts import ChatPromptTemplate
from langchain.memory import ConversationBufferMemory
# 設置 API 密鑰
os.environ["DASHSCOPE_API_KEY"] = 'YOUR_SECRET_KEY'
# 初始化通義千問模型
llm = ChatTongyi()
# 創(chuàng)建對話模板,包含多個變量(如歷史對話、當前問題和語氣)
prompt = ChatPromptTemplate.from_template('''
過往對話:
{history}
當前問題:
{current_question}
語氣:
{tone}
''')
# 構建對話鏈,包含模型、提示模板和對話記憶
conversation = LLMChain(
llm=llm,
prompt=prompt,
memory = ConversationBufferMemory(input_key="current_question"),
verbose=True
)
# 第一次對話:用戶告訴模型自己的名字,語氣為友好
input_question1 = "我是李劍鋒"
input_tone1 = "友好"
response_1 = conversation.invoke({"current_question": input_question1, "tone": input_tone1})
print("模型回答:", response_1["text"])
# 第二次對話:用戶詢問模型的身份,語氣為正式
input_question2 = "你是誰?"
input_tone2 = "正式"
response_2 = conversation.invoke({"current_question": input_question2, "tone": input_tone2})
print("模型回答:", response_2["text"])
# 第三次對話:用戶測試模型是否記得自己的名字,語氣為隨意
input_question3 = "你還記得我叫什么嗎?"
input_tone3 = "隨意"
response_3 = conversation.invoke({"current_question": input_question3, "tone": input_tone3})
print("模型回答:", response_3["text"])
如果我們希望像之前一樣,在對話中加入 SystemMessage(系統(tǒng)信息),在 LangChain 中實現(xiàn)這一功能其實非常簡單。
首先,我們需要從?langchain.prompts
?中導入?SystemMessagePromptTemplate?和?HumanMessagePromptTemplate。這是因為我們不再使用單一的?ChatPromptTemplate
,而是需要更細化地對?System?和?Human?兩部分的輸入分別進行設置:
from langchain.prompts import ChatPromptTemplate, SystemMessagePromptTemplate, HumanMessagePromptTemplate
接下來,利用?SystemMessagePromptTemplate?來定義系統(tǒng)角色(如希望模型扮演的身份或任務)。與此同時,將原來的提示詞模板改為?HumanMessagePromptTemplate?的格式,盡管直接使用?ChatPromptTemplate
?也可以完成基礎功能,但這種方式更靈活和模塊化。最后,通過?ChatPromptTemplate.from_messages
?將系統(tǒng)信息和用戶提示詞組合到一起。以下是示例代碼:
# 創(chuàng)建系統(tǒng)消息模板,用于設定模型的行為或角色
system_message = SystemMessagePromptTemplate.from_template("你是一個專業(yè)且友好的助手,請根據(jù)用戶的語氣調整回答風格。")
# 創(chuàng)建輸入信息,包含多個變量(如歷史對話、當前問題和語氣)
human_message = HumanMessagePromptTemplate.from_template('''
過往對話:
{history}
當前問題:
{current_question}
語氣:
{tone}
''')
# 合并系統(tǒng)消息和用戶消息到最終提示模板
prompt = ChatPromptTemplate.from_messages([system_message, human_message])
我們運行一下代碼就會發(fā)現(xiàn)SystemMessage確實加入進去了!并且調整后可以更加匹配我們的風格進行回復!這種方式讓我們能夠更加個性化地設計對話流,從而實現(xiàn)更精確的控制和更自然的交互效果。
> Entering new LLMChain chain...
Prompt after formatting:
System: 你是一個專業(yè)且友好的助手,請根據(jù)用戶的語氣調整回答風格。
Human:
過往對話:
當前問題:
我是李劍鋒
語氣:
友好
> Finished chain.
模型回答: 你好,李劍鋒!很高興見到你。有什么我可以幫你的嗎?
> Entering new LLMChain chain...
Prompt after formatting:
System: 你是一個專業(yè)且友好的助手,請根據(jù)用戶的語氣調整回答風格。
Human:
過往對話:
Human: 我是李劍鋒
AI: 你好,李劍鋒!很高興見到你。有什么我可以幫你的嗎?
當前問題:
你是誰?
語氣:
正式
> Finished chain.
模型回答: 我是李劍鋒的AI助手,負責提供信息和幫助解決問題。請問您需要了解什么?
> Entering new LLMChain chain...
Prompt after formatting:
System: 你是一個專業(yè)且友好的助手,請根據(jù)用戶的語氣調整回答風格。
Human:
過往對話:
Human: 我是李劍鋒
AI: 你好,李劍鋒!很高興見到你。有什么我可以幫你的嗎?
Human: 你是誰?
AI: 我是李劍鋒的AI助手,負責提供信息和幫助解決問題。請問您需要了解什么?
當前問題:
你還記得我叫什么嗎?
語氣:
隨意
> Finished chain.
模型回答: 當然記得,你是李劍鋒!有什么事情需要我?guī)兔Φ膯幔顒︿h?
這部分完整的代碼如下所示:
import os
from langchain_community.chat_models.tongyi import ChatTongyi
from langchain.chains import LLMChain
from langchain.prompts import ChatPromptTemplate, SystemMessagePromptTemplate, HumanMessagePromptTemplate
from langchain.memory import ConversationBufferMemory
# 設置 API 密鑰
os.environ["DASHSCOPE_API_KEY"] = 'YOUR_SECRET_KEY'
# 初始化通義千問模型
llm = ChatTongyi()
# 創(chuàng)建系統(tǒng)消息模板,用于設定模型的行為或角色
system_message = SystemMessagePromptTemplate.from_template("你是一個專業(yè)且友好的助手,請根據(jù)用戶的語氣調整回答風格。")
# 創(chuàng)建對話模板,包含多個變量(如歷史對話、當前問題和語氣)
human_message = HumanMessagePromptTemplate.from_template('''
過往對話:
{history}
當前問題:
{current_question}
語氣:
{tone}
''')
# 合并系統(tǒng)消息和用戶消息到最終提示模板
prompt = ChatPromptTemplate.from_messages([system_message, human_message])
# 構建對話鏈,包含模型、提示模板和對話記憶
conversation = LLMChain(
llm=llm,
prompt=prompt,
memory = ConversationBufferMemory(input_key="current_question"),
verbose=True
)
# 第一次對話:用戶告訴模型自己的名字,語氣為友好
input_question1 = "我是李劍鋒"
input_tone1 = "友好"
response_1 = conversation.invoke({"current_question": input_question1, "tone": input_tone1})
print("模型回答:", response_1["text"])
# 第二次對話:用戶詢問模型的身份,語氣為正式
input_question2 = "你是誰?"
input_tone2 = "正式"
response_2 = conversation.invoke({"current_question": input_question2, "tone": input_tone2})
print("模型回答:", response_2["text"])
# 第三次對話:用戶測試模型是否記得自己的名字,語氣為隨意
input_question3 = "你還記得我叫什么嗎?"
input_tone3 = "隨意"
response_3 = conversation.invoke({"current_question": input_question3, "tone": input_tone3})
print("模型回答:", response_3["text"])
相比于上節(jié)課學到的?ConversationChain,LLMChain?提供了更高的靈活性和擴展性,其核心價值在于對模型調用邏輯的精細化管理和模塊化設計。通過結合提示模板(Prompt Template)、記憶模塊(Memory)、以及多變量輸入等功能,LLMChain?能夠支持更復雜的場景,比如多輪對話、多任務鏈式調用、動態(tài)上下文傳遞等。而?ConversationChain?更適合快速實現(xiàn)基礎的對話功能,局限于單一的輸入和輸出邏輯。使用?LLMChain,開發(fā)者可以輕松構建結構化的交互流程,將模型的能力與特定場景需求結合,尤其在需要個性化輸出、多模塊集成(如記憶、數(shù)據(jù)庫、API 調用)時,LLMChain?顯得更加不可或缺。因此,LLMChain?是一個更加通用且可擴展的工具,適合復雜項目中更細致的邏輯實現(xiàn)和任務處理。
本文章轉載微信公眾號@機智流