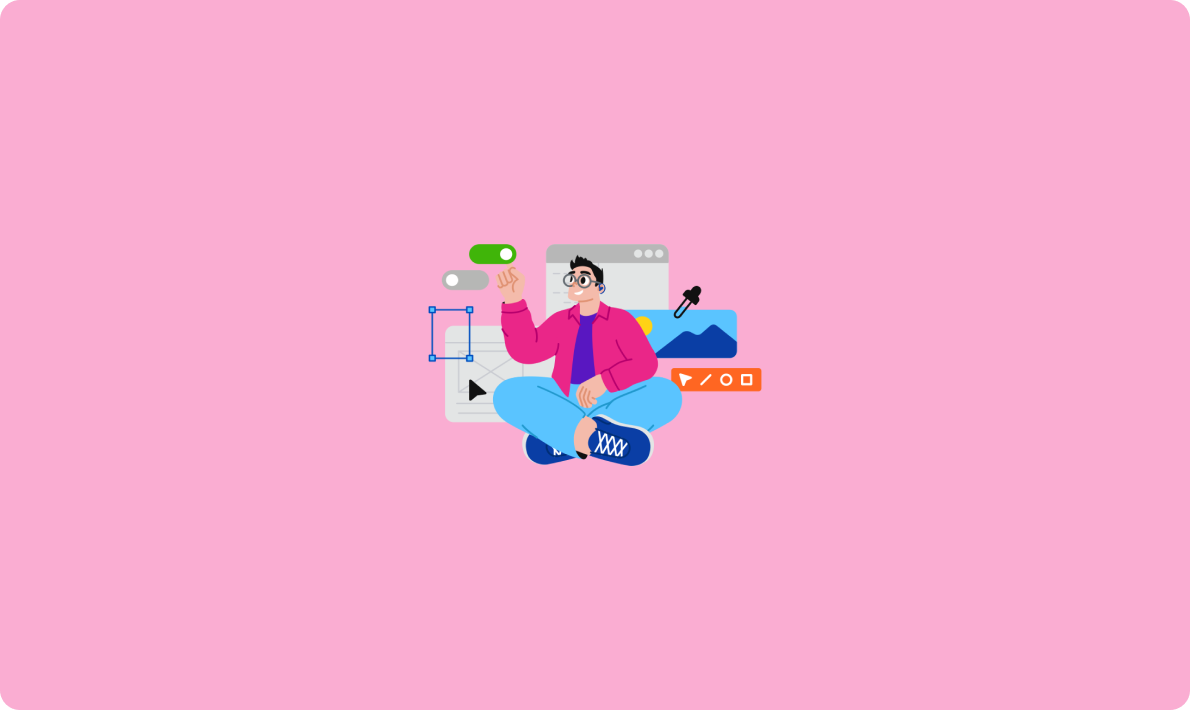
如何用AI進行情感分析
/root/share/install_conda_env_internlm_base.sh InternLM # 創建環境,默認pytorch 2.0.1 的環境
conda activate InternLM # 激活環境
安裝python依賴
# 升級pip
python -m pip install --upgrade pip
pip install modelscope==1.9.5
pip install transformers==4.35.2
pip install streamlit==1.24.0
pip install sentencepiece==0.1.99
pip install accelerate==0.24.1
pip install langchain==0.0.292
pip install gradio==4.4.0
pip install chromadb==0.4.15
pip install sentence-transformers==2.2.2
pip install unstructured==0.10.30
pip install markdown==3.3.7
模型下載
# 使用本地環境已有的模型
mkdir -p /root/data/model/Shanghai_AI_Laboratory
cp -r /root/share/temp/model_repos/internlm-chat-7b /root/data/model/Shanghai_AI_Laboratory/internlm-chat-7b
# 或者通過 ModelScope、HuggingFace 下載,如ModelScope
python -c "import torch; from modelscope import snapshot_download, AutoModel, AutoTokenizer; import os; model_dir = snapshot_download('Shanghai_AI_Laboratory/internlm-chat-7b', cache_dir='/root/data/model', revision='v1.0.3')"
詞向量模型下載
# 本次使用 huggingface_hub 下載 Sentence-Transformers
pip install -U huggingface_hub
python -c "import os; os.environ['HF_ENDPOINT'] = 'https://hf-mirror.com'; os.system('huggingface-cli download --resume-download sentence-transformers/paraphrase-multilingual-MiniLM-L12-v2 --local-dir /root/data/model/sentence-transformer')"
# 此處使用了 hf-mirror.com 鏡像網站
下載 NLTK 相關資源
# 避免眾所周知的原因下不到第三方庫資源
cd /root
git clone https://gitee.com/yzy0612/nltk_data.git --branch gh-pages
cd nltk_data
mv packages/* ./
cd tokenizers
unzip punkt.zip
cd ../taggers
unzip averaged_perceptron_tagger.zip
數據收集(以 InternLM 開源相關資料為例)
# 進入到數據庫盤
cd /root/data
# clone 上述開源倉庫
git clone --depth=1 https://github.com/InternLM/tutorial
git clone --depth=1 https://gitee.com/open-compass/opencompass.git
git clone --depth=1 https://gitee.com/InternLM/lmdeploy.git
git clone --depth=1 https://gitee.com/InternLM/xtuner.git
git clone --depth=1 https://gitee.com/InternLM/InternLM-XComposer.git
git clone --depth=1 https://gitee.com/InternLM/lagent.git
git clone --depth=1 https://gitee.com/InternLM/InternLM.git
我們遵循數據處理、LangChain 自定義LLM類構建(基于InternLM-Chat-7B)、Gradio 構建對話應用這個三個步驟來實現。后續我們構建自己的大模型應用也可以參照這種思路,只需替換不同的數據、不同的模型就是針對特定場景的大模型應用。完整代碼請參考:internlm-langchain-demo[5],代碼中注釋很完善,本文僅針對核心點講解。
在使用LangChain時,構建向量數據庫并且將加載的向量數據庫持久化到磁盤上的目的是可以提高語料庫的檢索效率和準確度。向量數據庫是一種利用向量空間模型來表示文檔的數據結構,它可以通過計算文檔向量之間的相似度來快速找出與用戶輸入相關的文檔片段。向量數據庫的構建過程需要對語料庫進行分詞、嵌入和索引等操作,這些操作比較耗時和資源,所以將構建好的向量數據庫保存到磁盤上,可以避免每次使用時都重復進行這些操作,從而節省時間和空間。加載向量數據庫時,只需要從磁盤上讀取已經構建好的向量數據庫,然后根據用戶輸入進行相似性檢索,就可以得到相關的文檔片段,再將這些文檔片段傳給LLM,得到最終的答案。這里我們使用的是開源向量數據庫Chroma[6],
# 首先導入所需第三方庫
# ...
from langchain.vectorstores import Chroma
from langchain.embeddings.huggingface import HuggingFaceEmbeddings
# ...
# 加載開源詞向量模型
embeddings = HuggingFaceEmbeddings(model_name="/root/data/model/sentence-transformer")
# 構建向量數據庫
# 定義持久化路徑
persist_directory = 'data_base/vector_db/chroma'
# 加載數據庫
vectordb = Chroma.from_documents(
documents=split_docs,
embedding=embeddings,
persist_directory=persist_directory
)
# 將加載的向量數據庫持久化到磁盤上
vectordb.persist()
我們只需執行一次?python create_db.py
即可創建向量數據庫。
LangChain 支持自定義 LLM,也就是我們常說的使用本地大模型。自定義 LLM 只需實現兩個必要條件:
_call
方法,用于接收一個字符串、一些可選的停止詞,并返回一個字符串。_llm_type
屬性。僅用于記錄日志。它還支持可選項:_identifying_params
?屬性,用于幫助打印自定義LLM類。# ...
from langchain.llms.base import LLM
from typing import Any, List, Optional
from langchain.callbacks.manager import CallbackManagerForLLMRun
# ...
class InternLM_LLM(LLM):
# 基于本地 InternLM 自定義 LLM 類
def _call(self, prompt : str, stop: Optional[List[str]] = None,
run_manager: Optional[CallbackManagerForLLMRun] = None,
**kwargs: Any):
# 重寫調用函數
system_prompt = """You are an AI assistant whose name is InternLM (書生·浦語).
- InternLM (書生·浦語) is a conversational language model that is developed by Shanghai AI Laboratory (上海人工智能實驗室). It is designed to be helpful, honest, and harmless.
- InternLM (書生·浦語) can understand and communicate fluently in the language chosen by the user such as English and 中文.
"""
messages = [(system_prompt, '')]
response, history = self.model.chat(self.tokenizer, prompt , history=messages)
return response
@property
def _llm_type(self) -> str:
return "InternLM"
不同版本的LangChain中自定義LLM類的具體實現代碼有些差別,可參考LangChain官方文檔:Custom LLM[7]
檢索問答鏈是LangChain的一個核心模塊,它可以根據用戶的查詢,在向量存儲庫中檢索相關文檔,并使用語言模型生成回答。要構建檢索問答鏈,我們需要以下幾個步驟:
# ...
from langchain.vectorstores import Chroma
from langchain.embeddings.huggingface import HuggingFaceEmbeddings
# ...
# 加載數據庫
vectordb = Chroma(
persist_directory=persist_directory, # 前文持久化路徑
embedding_function=embeddings
)
創建檢索QA鏈。我們可以使用LangChain提供的RetrievalQA模塊,或者自己定義鏈類型和參數。
from langchain.chains import RetrievalQA
qa_chain = RetrievalQA.from_chain_type(llm,retriever=vectordb.as_retriever(),return_source_documents=True,chain_type_kwargs={"prompt":QA_CHAIN_PROMPT})
創建 Gradio 應用:
#...
import gradio as gr
#...
block = gr.Blocks()
with block as demo:
#...
gr.close_all()
# 啟動新的 Gradio 應用,設置分享功能為 True,并使用環境變量 PORT1 指定服務器端口。
# demo.launch(share=True, server_port=int(os.environ['PORT1']))
# 直接啟動
demo.launch()
我們只需執行python run_gradio.py
即可運行部署一個我們專屬的知識庫。
本文章轉載微信公眾號@胡琦