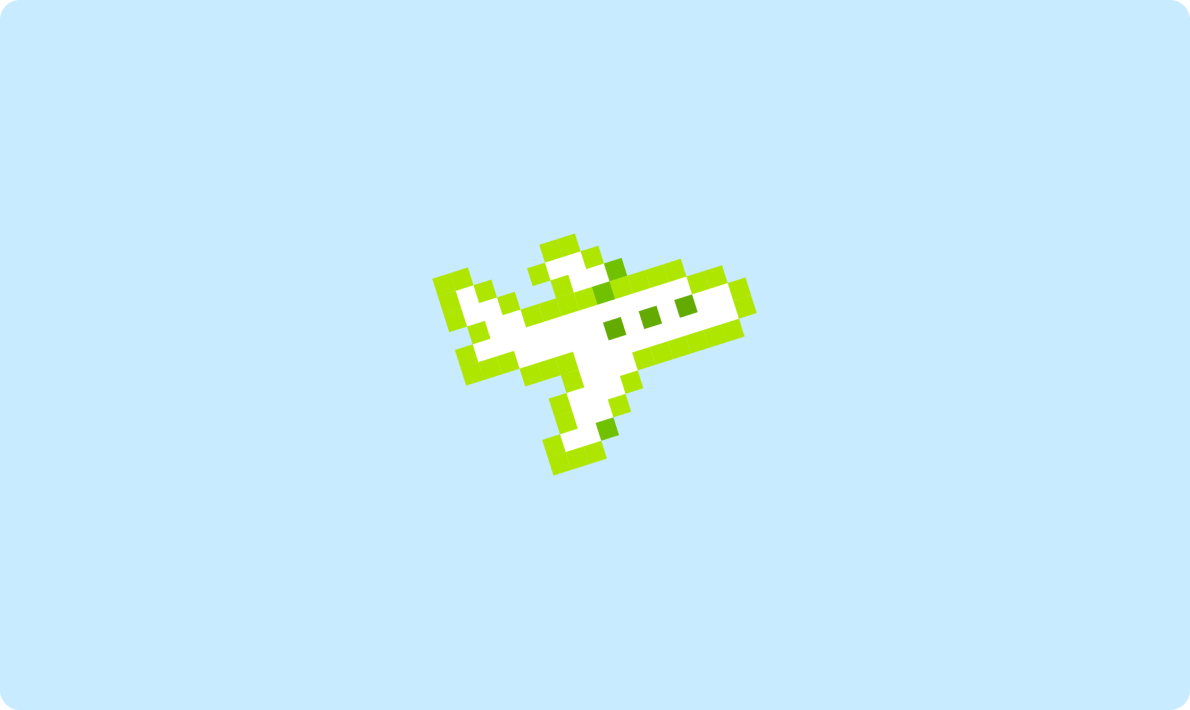
實時航班追蹤背后的技術:在線飛機追蹤器的工作原理
public abstract class AiModelStrategy {
/**
* SSE 流式對話
*/
public abstract void contextStreamChat(AiModeChatParams aiModeChatParams);
}
AiModeChatParams
的實現如下:
@Data
@NoArgsConstructor
public class AiModeChatParams {
@ApiModelProperty("用戶發送消息")
private String prompt;
@ApiModelProperty("會話 ID")
private String chatDialogueId;
@ApiModelProperty("模型選擇")
private String chatModel = ChatCompletion.Model.GPT_3_5_TURBO_16K.getName();
@ApiModelProperty(value = "對話機器人ID")
private String robotModelId;
@ApiModelProperty("最大 token 數量")
private int maxTokens = 2048;
@ApiModelProperty("密鑰集合")
private List<String> apiKeys = new ArrayList<>();
@ApiModelProperty("上下文消息")
private List<ChatMessage> chatMessageList;
@ApiModelProperty("請求路徑")
private String apiHost;
@ApiModelProperty("SSE")
private EventSourceListener eventSourceListener;
@ApiModelProperty("上傳圖片")
private List<String> imageList;
}
@Service
@Slf4j
public class AiModelFactory {
@Autowired
private Map<String, AiModelStrategy> map = new ConcurrentHashMap<>();
public AiModelStrategy getStrategy(String componentName) {
LogPrintUtils.info(log, "進入外部產品工廠方法 : {}", componentName);
AiModelStrategy strategy = map.get(componentName);
if (strategy == null) {
strategy = map.get("stdNullStrategy");
}
LogPrintUtils.info(log, "帶走了:{}", strategy);
return strategy;
}
}
@Service
public class AmXfxhStrategy extends AiModelStrategy {
@Override
public void contextStreamChat(AiModeChatParams aiModeChatParams) {
// 構建參數
// 發送請求
}
}
通過這種設計,我們可以將各個大模型的請求構建和發送邏輯放入策略工廠中,減少代碼重復,提高系統的可擴展性。
在對接大模型時,接口文檔是非常重要的參考資料。它描述了軟件組件或系統中的接口,包括接口名稱、方法、參數、返回值和異常等信息。通過接口文檔,我們可以了解如何調用大模型的 API。
以智譜 AI 的 GLM-4 模型為例,以下是通過 HTTP 調用該模型的示例代碼:
private static final String API_URL = ""; // 替換為實際的 API URL
private static final String API_KEY = ""; // 填寫您的 API Key
public static void main(String[] args) throws IOException {
OkHttpClient client = new OkHttpClient.Builder()
.connectTimeout(60, TimeUnit.SECONDS)
.writeTimeout(60, TimeUnit.SECONDS)
.readTimeout(60, TimeUnit.SECONDS)
.build();
JSONObject requestBodyJson = new JSONObject();
requestBodyJson.put("model", "glm-4-plus");
JSONArray messages = new JSONArray();
messages.put(new JSONObject().put("role", "system")
.put("content", "你是一個樂于解答各種問題的助手,你的任務是為用戶提供專業、準確、有見地的建議。"));
messages.put(new JSONObject().put("role", "user")
.put("content", "如何實現重定向"));
requestBodyJson.put("messages", messages);
RequestBody body = RequestBody.create(
requestBodyJson.toString(),
MediaType.parse("application/json")
);
Request request = new Request.Builder()
.url(API_URL)
.addHeader("Authorization", "Bearer " + API_KEY)
.post(body)
.build();
try (Response response = client.newCall(request).execute()) {
if (!response.isSuccessful()) {
throw new IOException("Unexpected code " + response);
}
String responseBody = response.body().string();
JSONObject jsonResponse = new JSONObject(responseBody);
String assistantReply = jsonResponse.getJSONArray("choices")
.getJSONObject(0)
.getJSONObject("message")
.getString("content");
System.out.println(assistantReply);
}
}
通過以上代碼,我們可以看到如何創建 HTTP 請求,發送請求并解析響應。
通過工廠策略模式,我們能夠高效地對接多家國內已備案的大模型,減少代碼重復,提升系統的可擴展性。同時,通過規范的接口文檔和 HTTP 調用,我們能夠更好地實現大模型的功能應用。這種方法不僅適用于 AI大模型,也可以應用于其他需要多策略、多接口對接的場景。