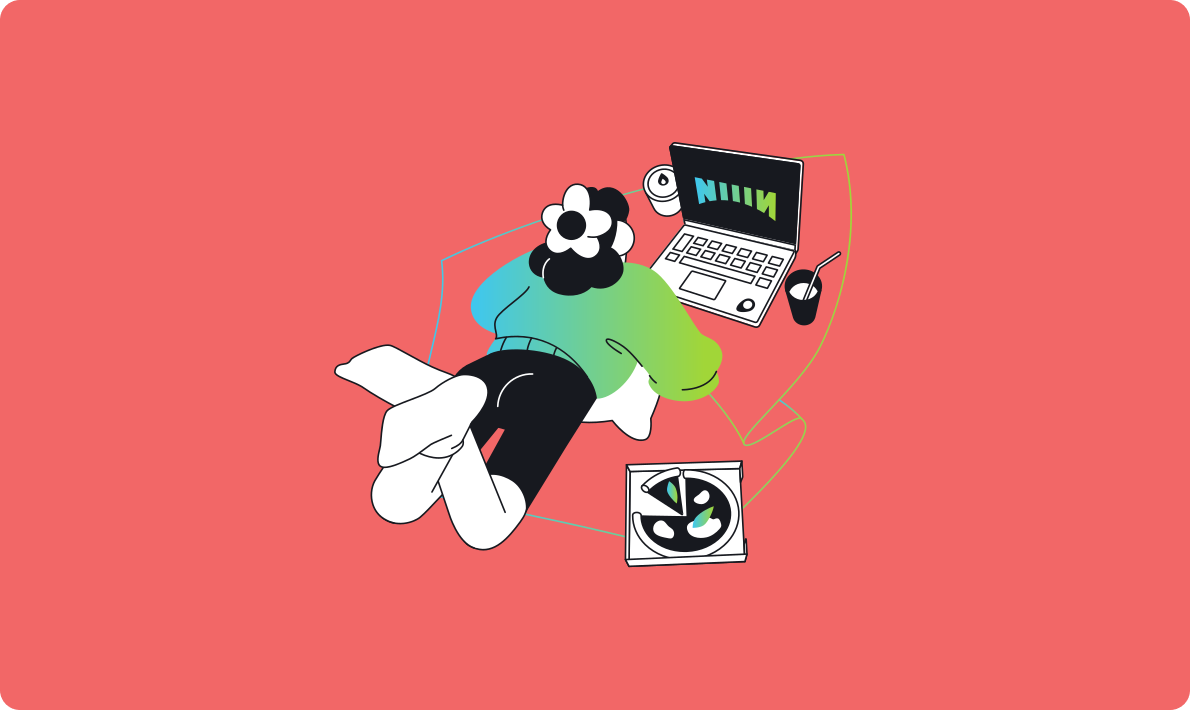
API可觀察性對(duì)于現(xiàn)代應(yīng)用程序的最大好處
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class IconSearchApiExample {
public static void main(String[] args) {
// API的URL
String apiUrl = "http://www.dlbhg.com/api/scd2024032538071845d417/search-icons-api-java-python-cpp";
// 創(chuàng)建HttpClient對(duì)象
HttpClient httpClient = HttpClients.createDefault();
// 創(chuàng)建HttpPost對(duì)象
HttpPost httpPost = new HttpPost(apiUrl);
// 設(shè)置請(qǐng)求體
StringEntity requestEntity = new StringEntity("{ \"query\": \"search term\" }", "UTF-8");
requestEntity.setContentType("application/json");
httpPost.setEntity(requestEntity);
try {
// 執(zhí)行請(qǐng)求
HttpResponse response = httpClient.execute(httpPost);
// 獲取響應(yīng)體
String responseBody = EntityUtils.toString(response.getEntity());
// 打印響應(yīng)
System.out.println(responseBody);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Python程序集成案例如下:
# 引入必要的庫(kù)
import requests
# API的URL
api_url = "http://www.dlbhg.com/api/scd2024032538071845d417/search-icons-api-java-python-cpp"
# 設(shè)置請(qǐng)求參數(shù)
data = {
"query": "search term"
}
# 發(fā)送POST請(qǐng)求
response = requests.post(api_url, json=data)
# 打印響應(yīng)內(nèi)容
print(response.text)
在C++中,使用curl
庫(kù)來發(fā)送HTTP POST請(qǐng)求。確保你的系統(tǒng)中已經(jīng)安裝了curl
庫(kù),然后使用以下代碼:
#include <iostream>
#include <curl/curl.h>
size_t writeCallback(char* contents, size_t size, size_t nmemb, void* userp) {
((std::string*)userp)->append((char*)contents, size * nmemb);
return size * nmemb;
}
int main() {
CURL* curl;
CURLcode res;
std::string readBuffer;
curl_global_init(CURL_GLOBAL_DEFAULT);
curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://www.dlbhg.com/api/scd2024032538071845d417/search-icons-api-java-python-cpp");
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "{\"query\":\"search term\"}");
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, writeCallback);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &readBuffer);
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
curl_global_cleanup();
std::cout << readBuffer << std::endl;
return 0;
}
確保在編譯時(shí)鏈接curl
庫(kù)。例如,使用g++編譯器,你可以使用以下命令:
g++ -o icon_search_api_example icon_search_api_example.cpp -lcurl
在上述代碼中,你需要將"search term"
替換為你的實(shí)際搜索查詢。同時(shí),確保你有權(quán)訪問開源圖標(biāo)搜索 API,并且已經(jīng)獲取了必要的授權(quán)信息(如果需要的話)。
在開發(fā)過程中,如果出于特定原因需要尋找替代方案,開源圖標(biāo)搜索API的替代品有很多。以下是一些替代方案,它們可能是商業(yè)服務(wù)、開源項(xiàng)目或者是其他API接口。
商業(yè)服務(wù)
開源項(xiàng)目
其他API接口
自建圖標(biāo)搜索系統(tǒng)
如果你有特定的需求,或者需要高度定制化的解決方案,可以考慮自己搭建圖標(biāo)搜索系統(tǒng)。這通常涉及到以下步驟:
示例代碼
以下是一個(gè)簡(jiǎn)化的Python代碼示例,展示了如何使用Elasticsearch來建立一個(gè)簡(jiǎn)單的圖標(biāo)搜索系統(tǒng):
from elasticsearch import Elasticsearch
# 初始化Elasticsearch客戶端
es = Elasticsearch()
# 索引圖標(biāo)數(shù)據(jù)
def index_icons(icon_data):
for icon in icon_data:
es.index(index="icons", body=icon)
# 搜索圖標(biāo)
def search_icons(query):
results = es.search(index="icons", body={"query": {"match": {"tags": query}}})
return results["hits"]["hits"]
# 示例數(shù)據(jù)
icon_data = [
{"name": "home", "tags": ["house", "residence"]},
{"name": "user", "tags": ["profile", "account"]},
# 更多圖標(biāo)數(shù)據(jù)...
]
# 索引數(shù)據(jù)
index_icons(icon_data)
# 搜索圖標(biāo)
search_results = search_icons("house")
print(search_results)
在這個(gè)例子中,我們使用Elasticsearch來索引圖標(biāo)數(shù)據(jù),并實(shí)現(xiàn)了一個(gè)基本的搜索功能。當(dāng)然,這只是一個(gè)起點(diǎn),實(shí)際的圖標(biāo)搜索系統(tǒng)可能需要考慮更多的因素,如圖標(biāo)的分類、顏色、風(fēng)格等。
冪簡(jiǎn)集成是國(guó)內(nèi)領(lǐng)先的API集成管理平臺(tái),專注于為開發(fā)者提供全面、高效、易用的API集成解決方案。冪簡(jiǎn)API平臺(tái)可以通過以下兩種方式找到所需API:通過關(guān)鍵詞搜索API(例如,輸入’人臉識(shí)別‘這類品類詞,更容易找到結(jié)果)、或者從API Hub分類頁進(jìn)入尋找。
此外,冪簡(jiǎn)集成博客會(huì)編寫API入門指南、多語言API對(duì)接指南、API測(cè)評(píng)等維度的文章,讓開發(fā)者快速使用目標(biāo)API。
API可觀察性對(duì)于現(xiàn)代應(yīng)用程序的最大好處
生成式AI及其對(duì)API和軟件開發(fā)的影響
2024年全球應(yīng)用程序編程接口(API)即服務(wù)市場(chǎng):現(xiàn)狀、趨勢(shì)及主要廠商分析
掌握編寫API文檔的方法:有效編寫 API文檔的技巧
API開發(fā)要點(diǎn)綜合指南
API貨幣化的最佳實(shí)踐:定價(jià)、打包和計(jì)費(fèi)
應(yīng)用程序開發(fā)中不可或缺的開放API
開發(fā)者生產(chǎn)力提升的API終極指南
制定藍(lán)圖:什么樣的API策略能夠確保未來的成功?
對(duì)比大模型API的內(nèi)容創(chuàng)意新穎性、情感共鳴力、商業(yè)轉(zhuǎn)化潛力
一鍵對(duì)比試用API 限時(shí)免費(fèi)