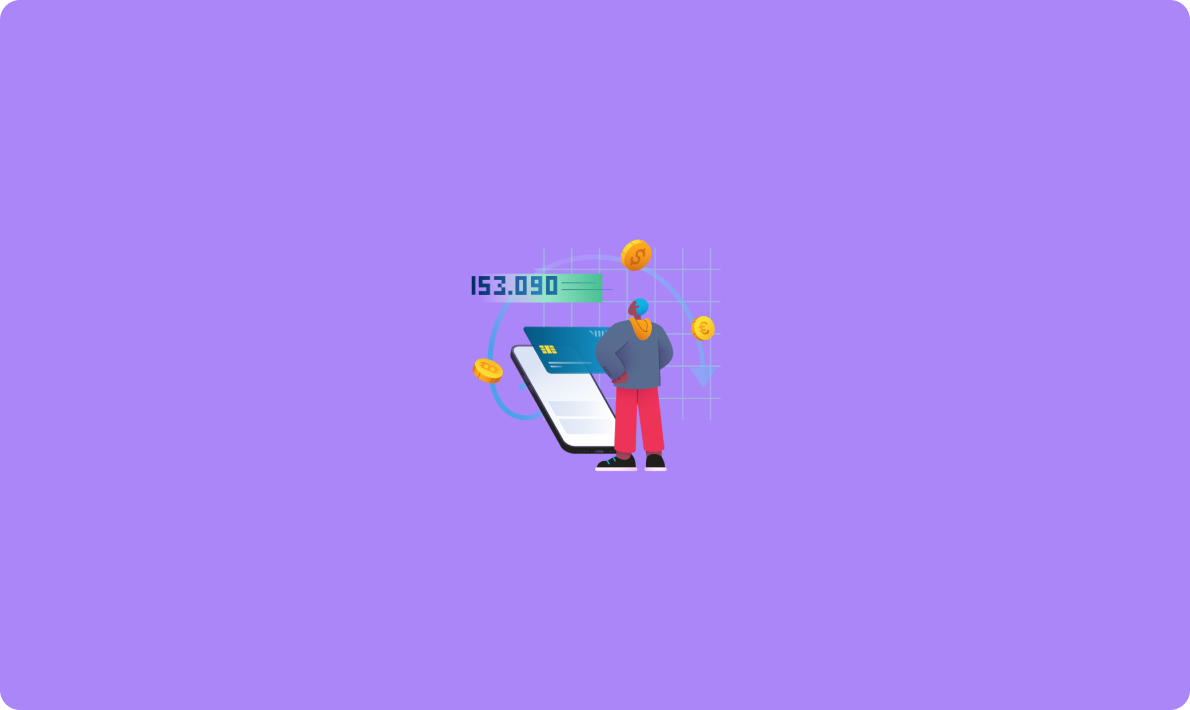
將 API 貨幣化:加速增長并減輕工程師的壓力
│
├── main.py
├── config.py
├── utils.py
├── requirements.txt
└── README.md
main.py
:主程序文件,用于啟動應(yīng)用和處理核心邏輯。config.py
:配置文件,用于存儲API的密鑰和其他設(shè)置。utils.py
:工具文件,包含一些輔助函數(shù),比如日期轉(zhuǎn)換和提醒功能。requirements.txt
:列出所有依賴包的文件。README.md
:項目說明文檔,介紹項目的用途和運行方法。為了實現(xiàn)節(jié)氣提醒助手,我們需要幾個第三方庫來簡化開發(fā)過程。主要的依賴包包括:
requests
:用于向 API 發(fā)送請求并處理響應(yīng)。schedule
:用于設(shè)置定時任務(wù),比如定時發(fā)送提醒。在 requirements.txt
文件中,我們需要列出這些依賴項。文件內(nèi)容如下:
requests==2.28.1
schedule==1.1.0
你可以通過以下命令安裝這些依賴:
pip install -r requirements.txt
下面是實現(xiàn)節(jié)氣提醒助手的核心代碼。我們將分為幾個部分來講解,包括獲取節(jié)氣數(shù)據(jù)、處理數(shù)據(jù)、設(shè)置定時提醒等。
config.py
在這個文件中,我們存儲 API 的基本信息和配置:
# config.py
API_URL = "http://api.explinks.com/v2/scd2023122597852d70c4d8/python-solar-term-reminder"
API_KEY = "your_api_key_here" # 替換為你自己的API密鑰
utils.py
這個文件包含了輔助函數(shù),例如獲取節(jié)氣信息和發(fā)送提醒:
# utils.py
import requests
from datetime import datetime
import json
def fetch_solar_terms(year):
response = requests.get(f"{API_URL}?year={year}", headers={"Authorization": f"Bearer {API_KEY}"})
if response.status_code == 200:
return response.json()
else:
raise Exception(f"API request failed with status code {response.status_code}")
def format_date(date_obj):
return f"公歷: {date_obj['gregorian_date']}, 農(nóng)歷: {date_obj['lunar_date']}, 屬相: {date_obj['zodiac']}"
def send_reminder(term_name, term_date):
print(f"提醒: {term_name} 將于 {term_date} 到來!")
main.py
主程序文件,負(fù)責(zé)啟動應(yīng)用并執(zhí)行主要邏輯:
# main.py
import schedule
import time
from datetime import datetime
from utils import fetch_solar_terms, send_reminder
def job():
year = datetime.now().year
terms = fetch_solar_terms(year)
for term in terms['solar_terms']:
term_name = term['name']
term_date = term['date']
send_reminder(term_name, term_date)
if __name__ == "__main__":
schedule.every().day.at("09:00").do(job) # 每天早上9點執(zhí)行任務(wù)
while True:
schedule.run_pending()
time.sleep(60)
要運行這個節(jié)氣提醒助手,你只需在項目根目錄下執(zhí)行以下命令:
python main.py
這會啟動應(yīng)用,每天早上 9 點自動檢查即將到來的節(jié)氣,并發(fā)送提醒。你可以根據(jù)需要調(diào)整提醒的時間和頻率。
通過上述步驟,我們成功地實現(xiàn)了一個節(jié)氣提醒助手,它利用 Python 語言和 二十四節(jié)氣 API 來提供精準(zhǔn)的節(jié)氣提醒。這個小項目不僅展示了如何將傳統(tǒng)文化與現(xiàn)代技術(shù)結(jié)合,還讓我們熟悉了如何使用 API 獲取數(shù)據(jù)并處理。希望你也能從中獲得樂趣,并嘗試在自己的項目中應(yīng)用這種方法。
如果你對 冪簡集成 提供的 API 感興趣,可以訪問 API 服務(wù)文檔 來獲取更多信息。通過這個平臺,你可以發(fā)現(xiàn)更多有趣的 API 服務(wù),助力你的開發(fā)工作,讓你的應(yīng)用更加智能和豐富。快來試試吧,讓你的代碼也能“節(jié)氣”盎然!