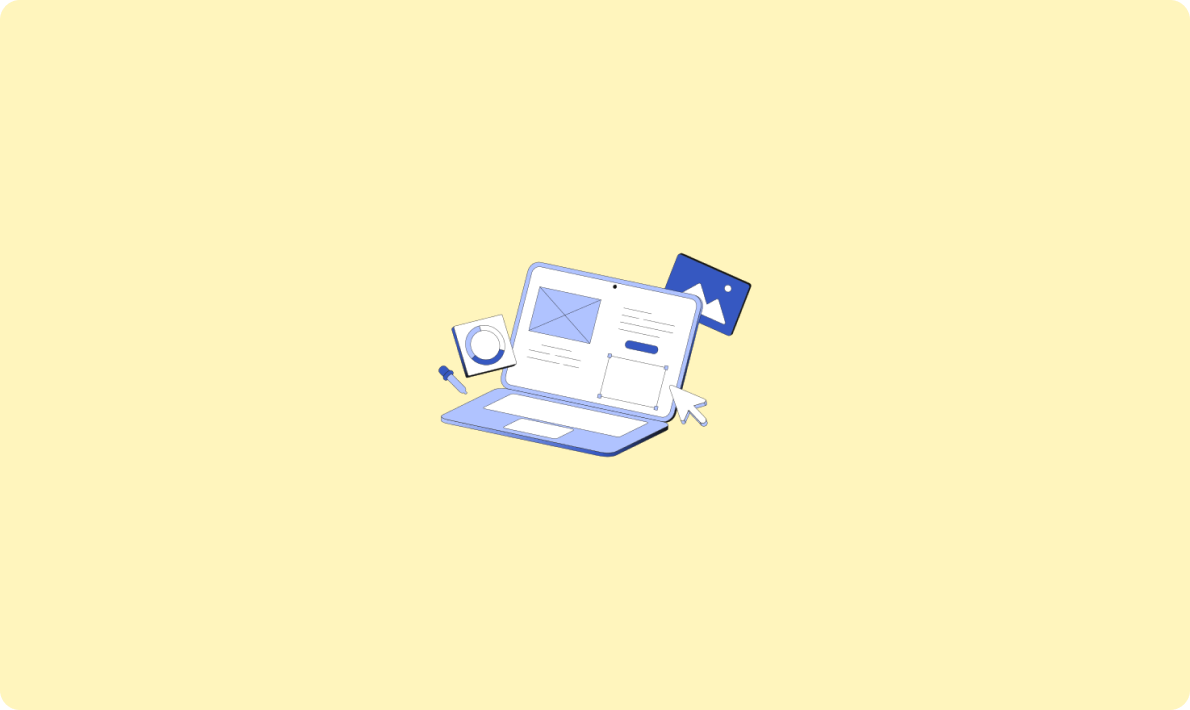
API優(yōu)先設(shè)計:構(gòu)建可擴展且靈活的軟件的現(xiàn)代方法
│
├── config/
│ └── config.json
│
├── src/
│ ├── __init__.py
│ ├── api_client.py
│ ├── contract_manager.py
│ └── utils.py
│
├── tests/
│ └── test_api_client.py
│
├── .gitignore
├── requirements.txt
└── main.py
config/
:存放配置文件,例如API密鑰和其他參數(shù)。src/
:主要的源代碼文件夾,包括API客戶端、合同管理和工具函數(shù)。tests/
:存放測試代碼,確保實現(xiàn)功能的正確性。.gitignore
:忽略文件配置,避免將不必要的文件提交到版本控制系統(tǒng)。requirements.txt
:記錄項目依賴的Python包。main.py
:主入口文件,負(fù)責(zé)啟動應(yīng)用程序。在實現(xiàn)項目之前,我們需要安裝一些Python包。你可以通過以下步驟來設(shè)置和安裝相關(guān)依賴:
python -m venv venv source venv/bin/activate # 在Windows上使用 venv\Scripts\activate
requirements.txt
文件,列出所需的依賴包: requests
pip
安裝這些依賴: pip install -r requirements.txt
requests
包是一個簡單易用的HTTP請求庫,用于與上上簽API進行交互。
以下是一個簡單的Python實現(xiàn)示例,展示如何使用上上簽API進行合同簽署。這個示例包括API客戶端的實現(xiàn)、合同管理和主入口文件。
api_client.py
:負(fù)責(zé)與上上簽API的交互。 import requests import json class APIClient: def __init__(self, api_key): self.api_key = api_key self.base_url = 'http://api.explinks.com/v2/scd20240403977721cf14a7/python-signature-api-automation' def post_contract(self, contract_data): headers = { 'Authorization': f'Bearer {self.api_key}', 'Content-Type': 'application/json' } response = requests.post(f'{self.base_url}/contracts', headers=headers, data=json.dumps(contract_data)) response.raise_for_status() return response.json() def get_status(self, contract_id): headers = { 'Authorization': f'Bearer {self.api_key}' } response = requests.get(f'{self.base_url}/contracts/{contract_id}', headers=headers) response.raise_for_status() return response.json()
contract_manager.py
:負(fù)責(zé)合同的管理邏輯。 from src.api_client import APIClient class ContractManager: def __init__(self, api_key): self.client = APIClient(api_key) def create_and_send_contract(self, contract_data): result = self.client.post_contract(contract_data) return result def check_contract_status(self, contract_id): status = self.client.get_status(contract_id) return status
main.py
:主入口文件,用于運行程序。 from src.contract_manager import ContractManager def main(): api_key = 'your_api_key_here' contract_data = { 'title': 'Sample Contract', 'content': 'This is a sample contract.', 'signers': ['signer@example.com'] } manager = ContractManager(api_key) response = manager.create_and_send_contract(contract_data) print('Contract sent:', response) contract_id = response.get('contract_id') if contract_id: status = manager.check_contract_status(contract_id) print('Contract status:', status) if __name__ == '__main__': main()
注意事項:
your_api_key_here
。contract_data
需要根據(jù)實際的API文檔進行調(diào)整。要啟動并運行項目,只需在項目根目錄下執(zhí)行以下命令:
python main.py
這將啟動主程序,創(chuàng)建并發(fā)送合同,并輸出合同的狀態(tài)。你可以根據(jù)實際需求對代碼進行微調(diào),以適應(yīng)你的具體應(yīng)用場景。
通過以上步驟,你應(yīng)該能夠使用Python和上上簽API實現(xiàn)自動合同簽署。這不僅能大幅度提高企業(yè)的合同處理效率,還能減少人工錯誤,提高合同管理的準(zhǔn)確性。在快速變化的商業(yè)環(huán)境中,自動化工具成為了提高生產(chǎn)力的關(guān)鍵。
推薦訪問冪簡集成API平臺,了解更多關(guān)于上上簽API的詳細信息和其他實用的API服務(wù)。無論你是開發(fā)者還是企業(yè)技術(shù)負(fù)責(zé)人,這個平臺都能為你提供強大的支持和資源,幫助你在技術(shù)創(chuàng)新的道路上邁出堅實的一步。