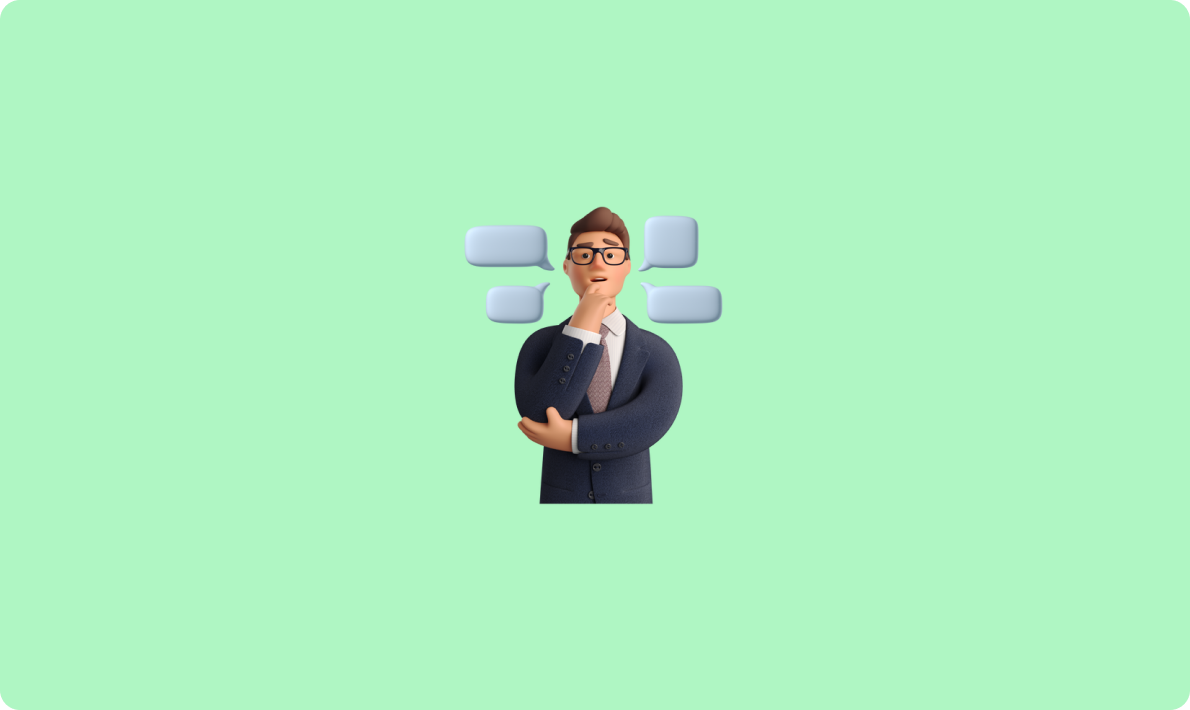
企業工商數據API用哪種?
首先,確保你已經安裝了Python環境和以下庫:
pip install qwen_agent requests #其它所需的模塊
這里如果沒有裝所需的庫,運行時會提示,根據提示安裝缺的庫即可。
沒有ollama也先去安裝ollama并運行qwen大模型,過程非常簡單,網上很多,不再贅述。
qwen智能體基本結構是這樣的:先定義工具類tools,然后定義智能體的任務描述,然后創建一個智能體,再然后就是web發布智能體服務,進行雙向通訊。
我們首先定義一個工具MobileAddress,用于查詢手機號的歸屬地。這個工具將使用一個外部API來獲取歸屬地信息。
@register_tool('get_mobile_address')
class MobileAddress(BaseTool):
description = '手機號歸屬地查詢服務,輸入手機號,返回該手機號的歸屬地。'
parameters = [{
'name': 'mobile',
'type': 'string',
'description': '輸入的手機號',
'required': True
}]
def call(self, params: str, **kwargs) -> str:
print("調用了function:", len(params))
print("字符串內容:",params)
try:
params_json = json.loads(params[:-1])
prompt = params_json["mobile"]
print("轉化后的號碼:", prompt)
except json.JSONDecodeError as e:
print("JSON解析錯誤:", e)
return "參數格式錯誤"
res=p.find(prompt)
print("原始查詢結果:",res)
return res
接下來,我們定義另一個工具WeatherByAddress,用于根據城市名稱查詢天氣信息。這個工具將使用另一個外部API來獲取天氣數據。
@register_tool('get_weather')
class WeatherByAddress(BaseTool):
description = '根據提供的城市名稱,查找代碼,并通過互聯網請求查詢天氣信息。'
parameters = [{'name': 'city', 'type': 'string', 'description': '城市名稱', 'required': True}]
def call(self, params: str, **kwargs) -> str:
try:
params_json = json.loads(params)
city_name = params_json["city"]
# 假設我們有一個城市代碼的映射字典
city_code = {'Beijing': '101010100'} # 示例代碼
url = f'https://www.weather.com.cn/weather1d/{city_code[city_name]}.shtml'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
match = re.search(r'var hour3data=(\{.*?\});', html_content)
if match:
hour3data = match.group(1)
return hour3data
else:
return "未找到天氣小時數據"
else:
return "請求失敗,狀態碼: {}".format(response.status_code)
except json.JSONDecodeError as e:
return "參數格式錯誤"
最后,我們創建一個Assistant實例,這個agent將使用我們定義的工具來處理用戶的輸入,并返回歸屬地和天氣信息。
from qwen_agent.agents import Assistant
# 配置LLM
llm_cfg = {
'model': 'qwen',#這里可以根據自己的大模型類型修改配置參數
'model_server': 'http://localhost:11434/v1',#這里可以根據自己的大模型類型修改配置參數
'generate_cfg': {'top_p': 0.8}
}
# 創建agent
system_instruction = '你扮演一個助手,會調用工具,首先獲取用戶輸入的手機號碼,并調用手機號歸屬地查詢服務工具獲得城市地址,然后再調用天氣查詢工具獲得所在城市的天氣信息,最后進行整理,輸出手機歸屬地和天氣信息'
tools = ['get_mobile_address', 'get_weather']
bot = Assistant(llm=llm_cfg, system_message=system_instruction, description='function calling', function_list=tools)
我們將使用FastAPI來創建一個簡單的Web界面,用戶可以通過這個界面輸入手機號,并獲取歸屬地和天氣信息。
from fastapi import FastAPI, Request, Form
from fastapi.responses import HTMLResponse
from fastapi.templating import Jinja2Templates
app = FastAPI()
templates = Jinja2Templates(directory="templates")
@app.get("/", response_class=HTMLResponse)
async def read_root(request: Request):
return templates.TemplateResponse("chat.html", {"request": request})
@app.post("/chat")
async def chat(message: str = Form(...)):
messages = [{'role': 'user', 'content': message}]
responses = bot.run(messages=messages)
return {"responses": [content['content'] for content in responses]}
# 運行FastAPI應用
if __name__ == '__main__':
import uvicorn
uvicorn.run(app, host='0.0.0.0', port=9000, workers=1)
創建一個簡單的html頁面,如下:
<!DOCTYPE html>
<html>
<head>
<title>Chat Interface</title>
<script>
function send_message() {
var message = document.getElementById("message").value;
if (message.trim() === "") {
alert("Message cannot be empty!");
return;
}
fetch("/chat", {
method: "POST",
headers: {
"Content-Type": "application/x-www-form-urlencoded",
},
body: "message=" + encodeURIComponent(message),
})
.then(response => {
if (!response.ok) {
throw new Error("Network response was not ok");
}
return response.json();
})
.then(data => {
var responses = data.responses;
var chat_window = document.getElementById("chat-window");
responses.forEach(response => {
var response_div = document.createElement("div");
response_div.innerText = response; // Fixed to access response directly
chat_window.appendChild(response_div);
});
document.getElementById("message").value = "";
chat_window.scrollTop = chat_window.scrollHeight;
})
.catch(error => console.error("Error:", error));
}
</script>
</head>
<body>
<div id="chat-window" style="width: 80%; height: 400px; border: 1px solid #000; overflow-y: scroll;"></div>
<input type="text" id="message" placeholder="Type a message..." style="height: 100px;width: 80%;">
<button onclick="send_message()" style="background-color: blue; color: white; font-size: larger; padding: 10px 20px;">Send</button>
</body>
</html>
至此,我們實現了一個anget,他可以接收我們輸入的電話號碼,并且調用本地大模型進行處理,先是調用一個手機號碼歸屬地查詢tool,再去調用一個天氣查詢爬蟲tool,最后大模型綜合tool的反饋信息進行整合后輸出給用戶。以上是簡單的實現,為了更加的準確好用需要進一步優化,包括qwen-anget本身好像有點問題,有時候只能調用一個手機號碼歸屬地函數發揮不是很穩定因此需要優化prompt,第二,可以加入更多檢查工具,比如,輸入的號碼檢查,讓大模型自己先檢查一下對不對,比如對回答進行一些過濾,過濾掉不必要的信息等。
本文章轉載微信公眾號@機智新語