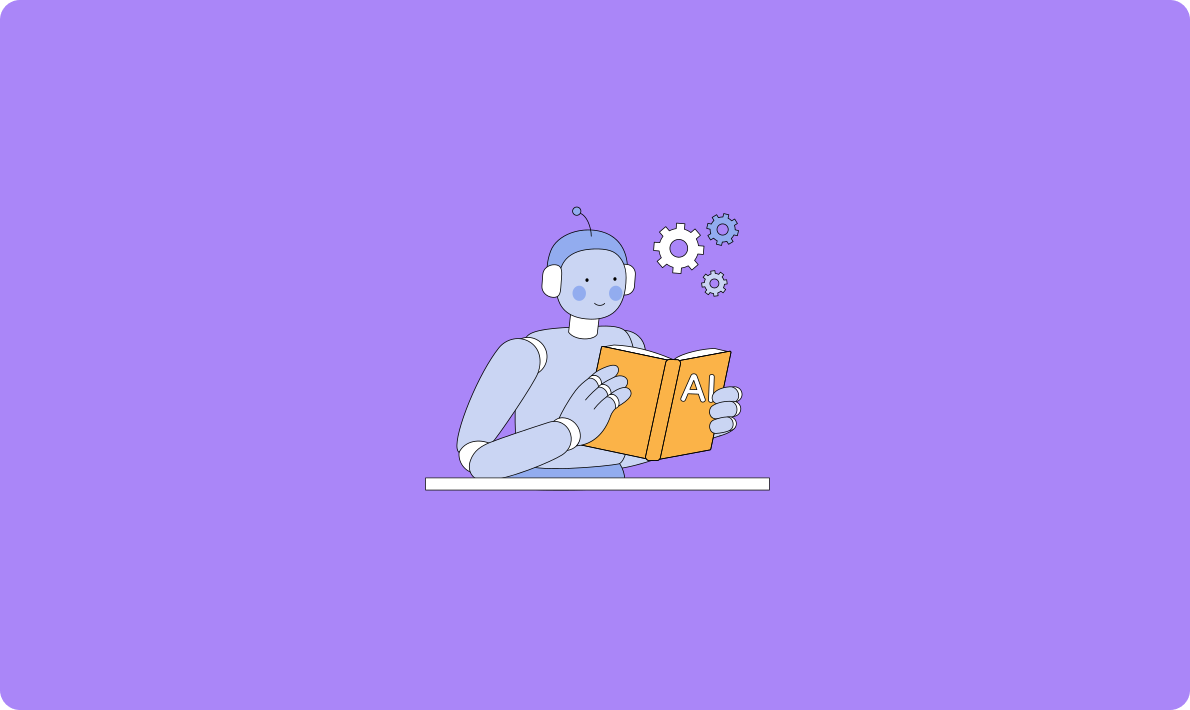
應(yīng)用程序開發(fā)中不可或缺的開放API
import java.net.HttpURLConnection;
import java.net.URL;
public class MoonshotAIAPIExample {
public static void main(String[] args) {
try {
String apiURL = "https://apihub.explinks.com//v2/scd2024052813171effabb6/chat"; // 假設(shè)的API路徑
String apiKey = "YOUR_API_KEY"; // 你的API密鑰
URL url = new URL(apiURL);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("Authorization", "Bearer " + apiKey);
// 發(fā)送POST請(qǐng)求
connection.connect();
// 這里可以添加要發(fā)送的數(shù)據(jù)
connection.getOutputStream().write(new byte[] {});
int responseCode = connection.getResponseCode();
System.out.println("Response Code: " + responseCode);
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// 打印結(jié)果
System.out.println(response.toString());
} catch (IOException e) {
e.printStackTrace();
}
}
}
<?php
$apiURL = "https://apihub.explinks.com//v2/scd2024052813171effabb6/chat"; // 假設(shè)的API路徑
$apiKey = "YOUR_API_KEY"; // 你的API密鑰
$data = array(); // 要發(fā)送的數(shù)據(jù)
$data_json = json_encode($data);
$ch = curl_init($apiURL);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($ch, CURLOPT_POSTFIELDS, $data_json);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json',
'Authorization: Bearer ' . $apiKey
));
$response = curl_exec($ch);
curl_close($ch);
// 打印結(jié)果
echo $response;
?>
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
var apiURL = "https://apihub.explinks.com//v2/scd2024052813171effabb6/chat"; // 假設(shè)的API路徑
var apiKey = "YOUR_API_KEY"; // 你的API密鑰
var client = new HttpClient();
var request = new HttpRequestMessage
{
Method = HttpMethod.Post,
RequestUri = new Uri(apiURL),
Content = new StringContent("", Encoding.UTF8, "application/json")
};
request.Headers.Add("Authorization", "Bearer " + apiKey);
// 發(fā)送POST請(qǐng)求
HttpResponseMessage response = await client.SendAsync(request);
string responseBody = await response.Content.ReadAsStringAsync();
// 打印結(jié)果
Console.WriteLine(responseBody);
}
}
對(duì)于尋找在線Moonshot AI大模型的替代服務(wù)商,可以試試這幾家:
對(duì)于尋找Moonshot AI的開源代碼替換解決方案,開發(fā)者可以考慮以下幾個(gè)流行的開源庫(kù):
假如你要使用并集成TensorFlow開發(fā)的開源機(jī)器學(xué)習(xí)庫(kù),用于構(gòu)建和訓(xùn)練一個(gè)簡(jiǎn)單的神經(jīng)網(wǎng)絡(luò)模型,下面是一個(gè)簡(jiǎn)單的示例幫助你理解集成流程。
首先,確保你已經(jīng)安裝了 TensorFlow。如果還沒(méi)有安裝,可以通過(guò) pip 安裝:
pip install tensorflow
然后,你可以使用以下 Python 代碼來(lái)創(chuàng)建一個(gè)簡(jiǎn)單的神經(jīng)網(wǎng)絡(luò):
import tensorflow as tf
# 定義輸入數(shù)據(jù)和標(biāo)簽
x_train = [1, 2, 3, 4]
y_train = [1, 2, 3, 4]
# 定義模型
model = tf.keras.models.Sequential([
tf.keras.layers.Dense(units=1, input_shape=[1])
])
# 編譯模型
model.compile(optimizer='sgd', loss='mean_squared_error')
# 訓(xùn)練模型
model.fit(x_train, y_train, epochs=50)
# 評(píng)估模型
loss = model.evaluate(x_train, y_train)
print(f'Loss: {loss}')
# 使用模型進(jìn)行預(yù)測(cè)
predictions = model.predict([5])
print(f'Predictions: {predictions}')
這段代碼首先導(dǎo)入 TensorFlow 庫(kù),然后定義了一些簡(jiǎn)單的訓(xùn)練數(shù)據(jù)。接著,創(chuàng)建了一個(gè)順序模型(Sequential),其中包含一個(gè)具有一個(gè)單元的密集(Dense)層。然后,編譯模型并指定優(yōu)化器和損失函數(shù)。之后,使用 fit
方法訓(xùn)練模型,并使用 evaluate
方法評(píng)估模型的性能。最后,使用 predict
方法進(jìn)行預(yù)測(cè)。
請(qǐng)注意,這只是一個(gè)非常基礎(chǔ)的例子,TensorFlow 提供了豐富的 API 來(lái)構(gòu)建更復(fù)雜的模型和執(zhí)行更高級(jí)的操作。
冪簡(jiǎn)集成是國(guó)內(nèi)領(lǐng)先的API平臺(tái),專注于為開發(fā)者提供全面、高效、易用的API集成解決方案。在冪簡(jiǎn)平臺(tái)可以通過(guò)以下兩種方式找到所需API:通過(guò)關(guān)鍵詞搜索API(例如,輸入’大模型‘這類品類詞,更容易找到結(jié)果)、或者從API Hub分類頁(yè)進(jìn)入尋找。
此外,冪簡(jiǎn)集成博客會(huì)編寫API入門指南、多語(yǔ)言API對(duì)接指南、API測(cè)評(píng)等維度的文章,讓開發(fā)者快速使用目標(biāo)API。
應(yīng)用程序開發(fā)中不可或缺的開放API
開發(fā)者生產(chǎn)力提升的API終極指南
制定藍(lán)圖:什么樣的API策略能夠確保未來(lái)的成功?
詳解API:應(yīng)用程序編程接口終極指南
精通API規(guī)范:構(gòu)建明確指導(dǎo)和預(yù)期的指南
API 優(yōu)先方法如何徹底改變軟件開發(fā)
掌握良好的 API 設(shè)計(jì)原則:是什么、為什么和怎么辦
API-first產(chǎn)品經(jīng)理的熱門 API 工具和 API 指標(biāo)
ChatGPT生態(tài)系統(tǒng)的安全漏洞導(dǎo)致第三方網(wǎng)站賬戶和敏感數(shù)據(jù)泄露
對(duì)比大模型API的內(nèi)容創(chuàng)意新穎性、情感共鳴力、商業(yè)轉(zhuǎn)化潛力
一鍵對(duì)比試用API 限時(shí)免費(fèi)