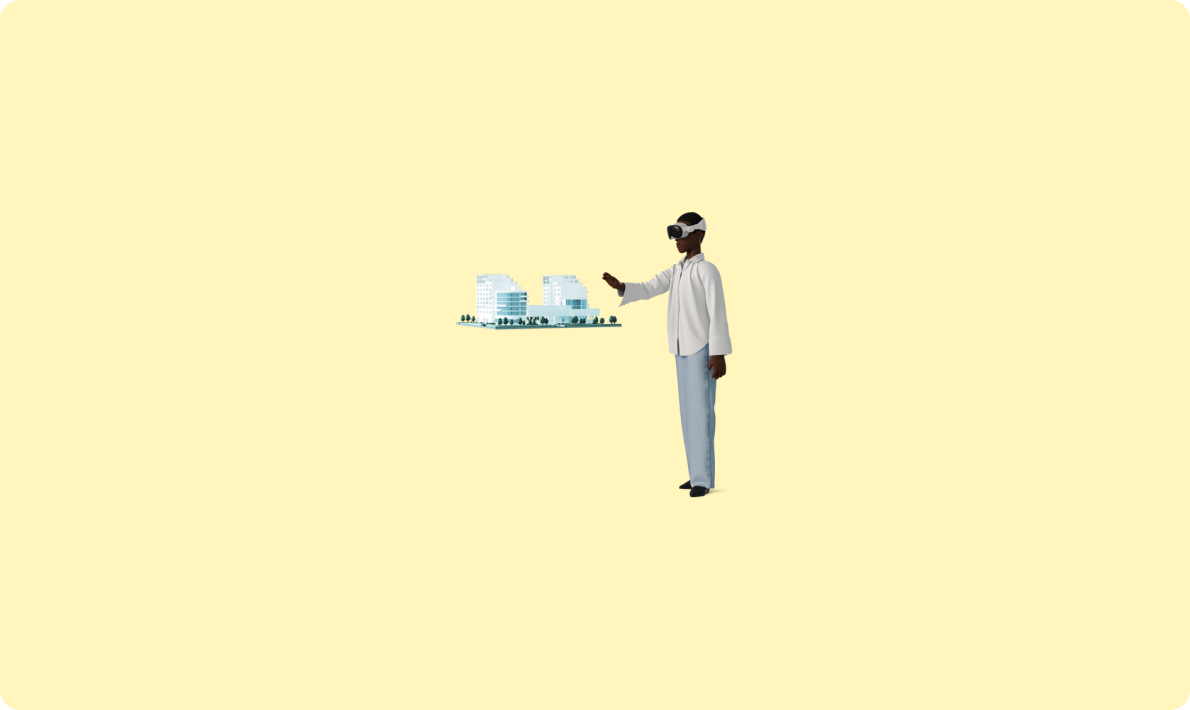
掌握API建模:基本概念和實踐
"name": "John Doe",
"email": "john.doe@example.com",
"age": 30
}
API的響應結構如下:
{
"status": "success",
"data": {
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com",
"age": 30
}
}
定義統一的錯誤響應格式:
{
"status": "error",
"message": "User not found"
}
在API開發流程中,開發階段是將設計轉化為實際代碼的關鍵步驟。我們使用Node.js和Express框架來實現這個API。
mkdir user-management-api
cd user-management-api
npm init -y
npm install express body-parser
創建app.js
文件,編寫API的核心邏輯:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
let users = [
{ id: 1, name: 'John Doe', email: 'john.doe@example.com', age: 30 },
{ id: 2, name: 'Jane Smith', email: 'jane.smith@example.com', age: 25 }
];
// 獲取用戶列表
app.get('/api/users', (req, res) => {
res.json({ status: 'success', data: users });
});
// 獲取單個用戶
app.get('/api/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).json({ status: 'error', message: 'User not found' });
res.json({ status: 'success', data: user });
});
// 創建新用戶
app.post('/api/users', (req, res) => {
const newUser = {
id: users.length + 1,
name: req.body.name,
email: req.body.email,
age: req.body.age
};
users.push(newUser);
res.status(201).json({ status: 'success', data: newUser });
});
// 更新用戶信息
app.put('/api/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).json({ status: 'error', message: 'User not found' });
user.name = req.body.name || user.name;
user.email = req.body.email || user.email;
user.age = req.body.age || user.age;
res.json({ status: 'success', data: user });
});
// 刪除用戶
app.delete('/api/users/:id', (req, res) => {
const userIndex = users.findIndex(u => u.id === parseInt(req.params.id));
if (userIndex === -1) return res.status(404).json({ status: 'error', message: 'User not found' });
users.splice(userIndex, 1);
res.json({ status: 'success', message: 'User deleted' });
});
const PORT = 3000;
app.listen(PORT, () => {
console.log(Server is running on http://localhost:${PORT}
);
});
node app.js
訪問http://localhost:3000/api/users
即可獲取用戶列表。
在API開發流程中,測試是確保API質量和穩定性的關鍵步驟。我們可以使用Postman或自動化測試工具進行測試。
GET http://localhost:3000/api/users
POST http://localhost:3000/api/users
,請求體為JSON格式的用戶數據。PUT http://localhost:3000/api/users/1
,請求體為更新的用戶數據。DELETE http://localhost:3000/api/users/1
使用Mocha和Chai編寫單元測試:
npm install mocha chai chai-http --save-dev
創建test/test.js
文件:
const chai = require('chai');
const chaiHttp = require('chai-http');
const app = require('../app');
chai.use(chaiHttp);
const expect = chai.expect;
describe('User API', () => {
it('should return all users', (done) => {
chai.request(app)
.get('/api/users')
.end((err, res) => {
expect(res).to.have.status(200);
expect(res.body.data).to.be.an('array');
done();
});
});
it('should create a new user', (done) => {
chai.request(app)
.post('/api/users')
.send({ name: 'Alice', email: 'alice@example.com', age: 28 })
.end((err, res) => {
expect(res).to.have.status(201);
expect(res.body.data.name).to.equal('Alice');
done();
});
});
});
運行測試:
npx mocha
在API開發流程中,部署是將API發布到生產環境的關鍵步驟。以下是使用Docker部署的示例:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["node", "app.js"]
docker build -t user-management-api .
docker run -p 3000:3000 user-management-api
在API開發流程中,維護是確保API長期穩定運行的關鍵步驟。
使用工具如Prometheus和Grafana監控API的性能指標。
收集用戶的反饋和建議,持續改進API的功能和用戶體驗。
根據用戶需求和市場變化,定期更新API的功能和性能。
本文詳細介紹了API開發流程,并提供了代碼示例幫助理解。通過需求分析、設計、開發、測試、部署和維護等環節,可以開發出高質量、高性能的API。希望本文能夠為你的API開發流程提供有價值的參考和指導。