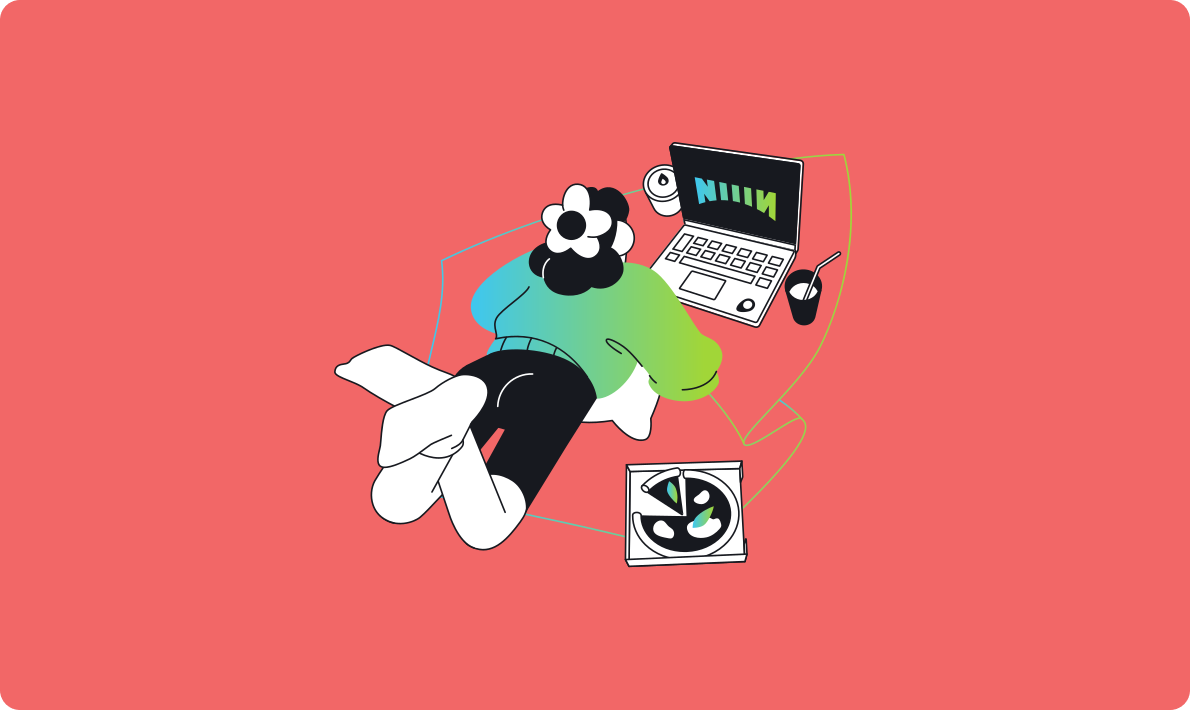
API可觀察性對于現代應用程序的最大好處
// 替換為實際的API訪問密鑰
$apiKey = 'YOUR_API_KEY';
$perPage = 15; // 每頁顯示的圖片數量
$page = 1; // 當前頁碼
// 構建請求URL
$url = 'https://api.pexels.com/v1/curated?per_page=' . $perPage . '&page=' . $page;
// 初始化curl
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Authorization: ' . $apiKey
));
// 執行curl請求并獲取響應
$response = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
// 檢查HTTP響應狀態碼
if ($httpCode == 200) {
// 解析JSON響應
$photos = json_decode($response, true);
// 輸出圖片列表
foreach ($photos['photos'] as $photo) {
echo 'Photo ID: ' . $photo['id'] . '<br>';
echo 'Photo URL: ' . $photo['src']['original'] . '<br>';
echo 'Photo Photographer: ' . $photo['photographer'] . '<br>';
echo 'Photo Photographer URL: ' . $photo['photographer_url'] . '<br>';
echo '<hr>';
}
} else {
echo 'Error: ' . $httpCode . '<br>';
}
?>
在C++中,你可以使用libcurl
庫來發送HTTP請求。下面是一個簡單的C++示例,展示了如何使用libcurl來獲取Pexels圖片庫中的圖片列表。
#include <iostream>
#include <curl/curl.h>
#include <sstream>
#include <string>
// 回調函數,用于處理HTTP響應數據
size_t writeCallback(char* contents, size_t size, size_t nmemb, void* userp) {
((std::stringstream*)userp)->write(contents, size * nmemb);
return size * nmemb;
}
int main(void) {
CURL* curl;
CURLcode res;
std::stringstream response;
std::string apiKey = "YOUR_API_KEY"; // 替換為實際的API訪問密鑰
std::string perPage = "15"; // 每頁顯示的圖片數量
std::string page = "1"; // 當前頁碼
std::string url = "https://api.pexels.com/v1/curated?per_page=" + perPage + "&page=" + page;
curl_global_init(CURL_GLOBAL_DEFAULT);
curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_URL, url.c_str());
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, writeCallback);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &response);
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, curl_slist_append(NULL, ("Authorization: " + apiKey).c_str()));
res = curl_easy_perform(curl);
if (res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
} else {
// 這里可以解析JSON響應并輸出圖片信息
std::string jsonResponse = response.str();
// ...
}
/* always cleanup */
curl_easy_cleanup(curl);
}
curl_global_cleanup();
return 0;
}
請確保在使用上述代碼之前,你已經安裝了libcurl庫,并且在你的C++項目中正確配置了libcurl。你需要將YOUR_API_KEY
替換為你的Pexels API訪問密鑰。
當然,除了Pexels圖片庫API之外,還有一些其他的免費和付費圖片庫API可以作為替代方案。以下是一些流行的圖片庫API以及它們的一些特點:
選擇哪個API取決于你的具體需求,比如圖片的質量、數量、版權狀況以及你的預算。如果你需要大量的高質量圖片并且不介意付費,那么像Shutterstock或Getty Images這樣的服務可能是更好的選擇。如果你正在尋找免費資源,那么Pexels、Unsplash、Pixabay和StockSnap.io都是不錯的選擇。
在選擇API時,務必仔細閱讀其服務條款和使用限制,以確保你的應用符合其要求,并且不會因為違反條款而導致服務中斷。
冪簡集成是國內領先的API集成管理平臺,專注于為開發者提供全面、高效、易用的API集成解決方案。冪簡API平臺可以通過以下兩種方式找到所需API:通過關鍵詞搜索API(例如,輸入’人臉識別‘這類品類詞,更容易找到結果)、或者從API Hub分類頁進入尋找。
此外,冪簡集成博客會編寫API入門指南、多語言API對接指南、API測評等維度的文章,讓開發者快速使用目標API。