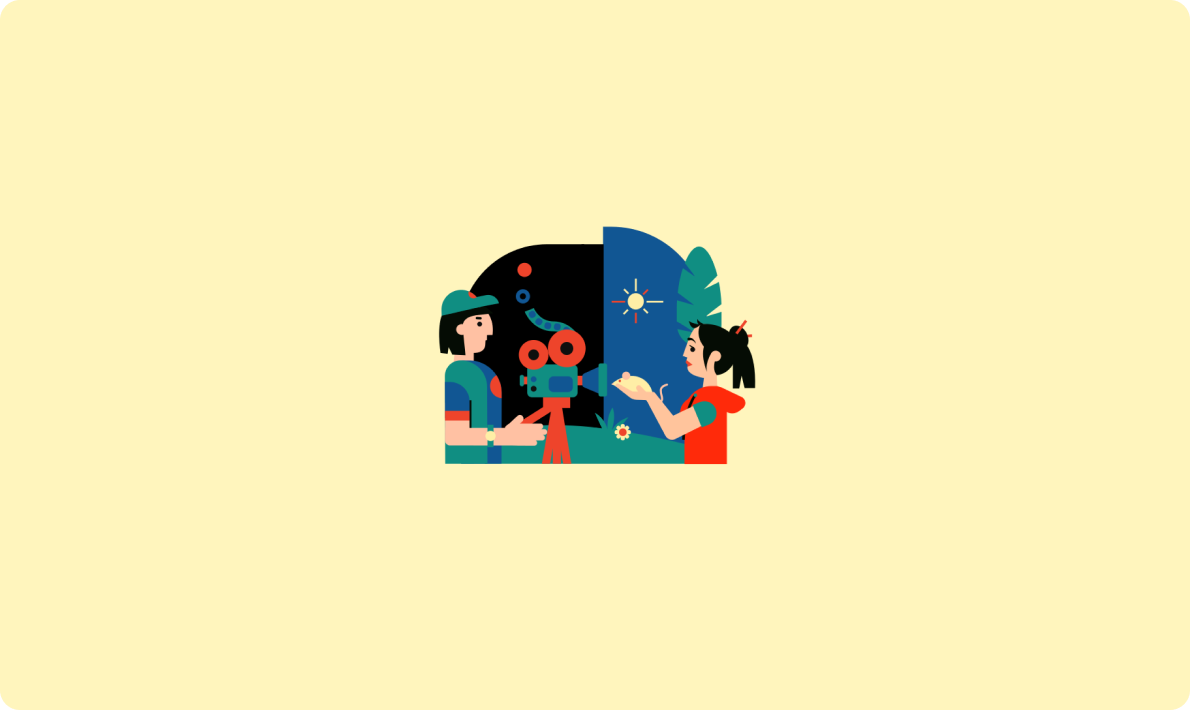
有哪些新聞媒體提供Open API?
API 響應通常包含生成的文本、請求狀態等信息。你可以通過解析響應對象來獲取所需的數據。
# 獲取生成的文本
generated_text = response.choices[0].text.strip()
# 獲取請求 ID
request_id = response.id
# 獲取請求狀態
status = response.status
利用 OpenAI的實時 API,你可以輕松構建一個實時聊天機器人。以下是一個簡單的示例:
import openai
openai.api_key = 'your-api-key'
def chat_with_bot(prompt):
response = openai.Completion.create(
engine="text-davinci-003",
prompt=prompt,
max_tokens=150
)
return response.choices[0].text.strip()
while True:
user_input = input("You: ")
if user_input.lower() in ["exit", "quit"]:
break
bot_response = chat_with_bot(user_input)
print(f"Bot: {bot_response}")
OpenAI 的實時 API 還可以用于代碼補全。以下是一個示例,展示如何使用 API 補全 Python代碼:
import openai
openai.api_key = 'your-api-key'
def complete_code(prompt):
response = openai.Completion.create(
engine="code-davinci-002",
prompt=prompt,
max_tokens=100
)
return response.choices[0].text.strip()
code_prompt = """
def fibonacci(n):
if n <= 0:
return 0
elif n == 1:
return 1
else:
return fibonacci(n-1) + fibonacci(n-2)
"""
completed_code = complete_code(code_prompt)
print(completed_code)
為了減少延遲并提高吞吐量,建議將多個請求合并為一個批量請求。以下是一個示例:
import openai
openai.api_key = 'your-api-key'
prompts = [
"Translate the following English text to French: 'Hello, how are you?'",
"Write a short story about a robot learning to love.",
"Explain the concept of quantum computing in simple terms."
]
responses = openai.Completion.create(
engine="text-davinci-003",
prompt=prompts,
max_tokens=60
)
for i, response in enumerate(responses.choices):
print(f"Response {i+1}: {response.text.strip()}")
在實際應用中,網絡波動或 API 限流可能導致請求失敗。建議實現錯誤處理與重試機制,以提高系統的穩定性。
import openai
import time
openai.api_key = 'your-api-key'
def safe_api_call(prompt, retries=3):
for i in range(retries):
try:
response = openai.Completion.create(
engine="text-davinci-003",
prompt=prompt,
max_tokens=60
)
return response.choices[0].text.strip()
except Exception as e:
print(f"Attempt {i+1} failed: {e}")
time.sleep(2 ** i) # 指數退避
return "API call failed after retries."
result = safe_api_call("Translate the following English text to French: 'Hello, how are you?'")
print(result)
OpenAI 的實時 API 為開發者提供了強大的工具,能夠輕松實現自然語言處理、代碼補全等功能。通過本文的介紹,你應該已經掌握了如何使用 OpenAI 的實時 API,并了解了其在實際項目中的應用。希望這些內容能夠幫助你在開發過程中更好地利用 OpenAI 的技術,構建出更加智能的應用。