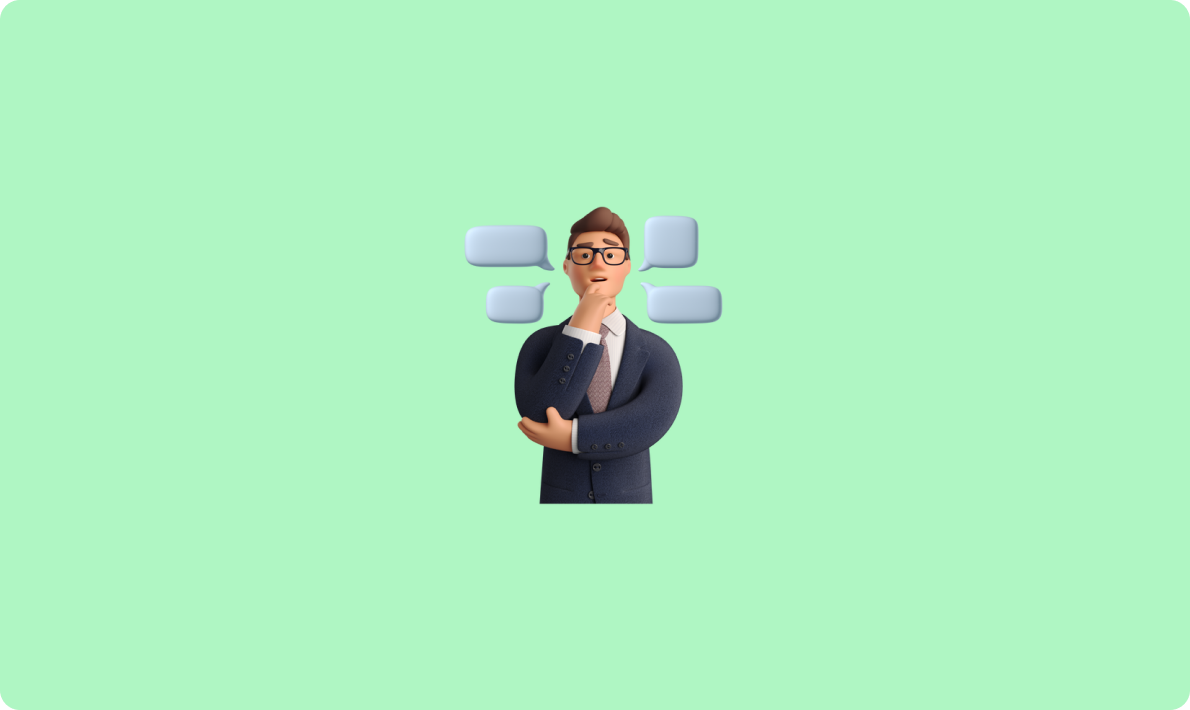
企業工商數據API用哪種?
如果你尚未登錄或注冊,會自動跳轉到登錄頁面邀請您來注冊和登錄,登錄注冊之后會自動返回當前頁面。
在首次申請時會有免費額度贈送,可以免費使用該 API。
首先先了解下基本的使用方式,就是輸入問題,獲得回答,只需要簡單地傳遞一個 question
字段,并指定相應模型即可。
比如說詢問:“What’s your name?”,我們接下來就可以在界面上填寫對應的內容,如圖所示:
可以看到這里我們設置了 Request Headers,包括:
accept
:想要接收怎樣格式的響應結果,這里填寫為 application/json
,即 JSON 格式。authorization
:調用 API 的密鑰,申請之后可以直接下拉選擇。另外設置了 Request Body,包括:
model
:模型的選擇,比如主流的 GPT 3.5,GPT 4 等。question
:需要詢問的問題,可以是任意的純文本。選擇之后,可以發現右側也生成了對應代碼,如圖所示:
點擊「Try」按鈕即可進行測試,如上圖所示,這里我們就得到了如下結果:
{
"answer": "I am an AI language model developed by OpenAI and I don't have a personal name. However, you can call me GPT or simply Chatbot. How can I assist you today?"
}
可以看到,這里返回的結果中有一個 answer
字段,就是該問題的回答。我們可以輸入任意問題,就可以得到任意的回答。
如果你不需要任何多輪對話的支持,這個 API 可以極大方便你的對接。
另外如果想生成對應的對接代碼,可以直接復制生成,例如 CURL 的代碼如下:
curl -X POST 'https://api.acedata.cloud/aichat/conversations' \
-H 'accept: application/json' \
-H 'authorization: Bearer {token}' \
-H 'content-type: application/json' \
-d '{
"model": "gpt-3.5",
"question": "What's your name?"
}'
Python 的對接代碼如下:import requests
?
url = "https://api.acedata.cloud/aichat/conversations"
?
headers = {
"accept": "application/json",
"authorization": "Bearer {token}",
"content-type": "application/json"
}
?
payload = {
"model": "gpt-3.5",
"question": "What's your name?"
}
?
response = requests.post(url, json=payload, headers=headers)
print(response.text)
如果您想要對接多輪對話功能,需要傳遞一個額外參數 stateful
,其值為 true
,后續的每次請求都要攜帶該參數。傳遞了 stateful
參數之后,API 會額外返回一個 id
參數,代表當前對話的 ID,后續我們只需要將該 ID 作為參數傳遞,就可以輕松實現多輪對話。
下面我們來演示下具體的操作。
第一次請求,將 stateful
參數設置為 true
,并正常傳遞 model
和 question
參數,如圖所示:
對應代碼如下:
curl -X POST 'https://api.acedata.cloud/aichat/conversations' \
-H 'accept: application/json' \
-H 'authorization: Bearer {token}' \
-H 'content-type: application/json' \
-d '{
"model": "gpt-3.5",
"question": "What's your name?",
"stateful": true
}'
可以得到如下回答:
{
"answer": "I am an AI language model created by OpenAI and I don't have a personal name. You can simply call me OpenAI or ChatGPT. How can I assist you today?",
"id": "7cdb293b-2267-4979-a1ec-48d9ad149916"
}
第二次請求,將第一次請求返回的 id
字段作為參數傳遞,同時 stateful
參數依然設置為 true
,詢問「What I asked you just now?」,如圖所示:
對應代碼如下:
curl -X POST 'https://api.acedata.cloud/aichat/conversations' \
-H 'accept: application/json' \
-H 'authorization: Bearer {token}' \
-H 'content-type: application/json' \
-d '{
"model": "gpt-3.5",
"stateful": true,
"id": "7cdb293b-2267-4979-a1ec-48d9ad149916",
"question": "What I asked you just now?"
}'
結果如下:
{
"answer": "You asked me what my name is. As an AI language model, I do not possess a personal identity, so I don't have a specific name. However, you can refer to me as OpenAI or ChatGPT, the names used for this AI model. Is there anything else I can help you with?",
"id": "7cdb293b-2267-4979-a1ec-48d9ad149916"
}
可以看到,就可以根據上下文回答對應的問題了。
該接口也支持流式響應,這對網頁對接十分有用,可以讓網頁實現逐字顯示效果。
如果想流式返回響應,可以更改請求頭里面的 accept
參數,修改為 application/x-ndjson
。
修改如圖所示,不過調用代碼需要有對應的更改才能支持流式響應。
將?accept
?修改為?application/x-ndjson
?之后,API 將逐行返回對應的 JSON 數據,在代碼層面我們需要做相應的修改來獲得逐行的結果。
Python 樣例調用代碼:
import requests
url = "https://api.acedata.cloud/aichat/conversations"
headers = {
"accept": "application/x-ndjson",
"authorization": "Bearer {token}",
"content-type": "application/json"
}
payload = {
"model": "gpt-3.5",
"stateful": True,
"id": "7cdb293b-2267-4979-a1ec-48d9ad149916",
"question": "Hello"
}
response = requests.post(url, json=payload, headers=headers, stream=True)
for line in response.iter_lines():
print(line.decode())
輸出效果如下:
{"answer": "Hello", "delta_answer": "Hello", "id": "7cdb293b-2267-4979-a1ec-48d9ad149916"}
{"answer": "Hello!", "delta_answer": "!", "id": "7cdb293b-2267-4979-a1ec-48d9ad149916"}
{"answer": "Hello! How", "delta_answer": " How", "id": "7cdb293b-2267-4979-a1ec-48d9ad149916"}
{"answer": "Hello! How can", "delta_answer": " can", "id": "7cdb293b-2267-4979-a1ec-48d9ad149916"}
{"answer": "Hello! How can I", "delta_answer": " I", "id": "7cdb293b-2267-4979-a1ec-48d9ad149916"}
{"answer": "Hello! How can I assist", "delta_answer": " assist", "id": "7cdb293b-2267-4979-a1ec-48d9ad149916"}
{"answer": "Hello! How can I assist you", "delta_answer": " you", "id": "7cdb293b-2267-4979-a1ec-48d9ad149916"}
{"answer": "Hello! How can I assist you today", "delta_answer": " today", "id": "7cdb293b-2267-4979-a1ec-48d9ad149916"}
{"answer": "Hello! How can I assist you today?", "delta_answer": "?", "id": "7cdb293b-2267-4979-a1ec-48d9ad149916"}
可以看到,響應里面的 answer
即為最新的回答內容,delta_answer
則是新增的回答內容,您可以根據結果來對接到您的系統中。
JavaScript 也是支持的,比如 Node.js 的流式調用代碼如下:
const axios = require("axios");
const url = "https://api.acedata.cloud/aichat/conversations";
const headers = {
"Content-Type": "application/json",
Accept: "application/x-ndjson",
Authorization: "Bearer {token}",
};
const body = {
question: "Hello",
model: "gpt-3.5",
stateful: true,
};
axios
.post(url, body, { headers: headers, responseType: "stream" })
.then((response) => {
console.log(response.status);
response.data.on("data", (chunk) => {
console.log(chunk.toString());
});
})
.catch((error) => {
console.error(error);
});
Java 樣例代碼:
String url = "https://api.acedata.cloud/aichat/conversations";
OkHttpClient client = new OkHttpClient();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\"question\": \"Hello\", \"stateful\": true, \"model\": \"gpt-3.5\"}");
Request request = new Request.Builder()
.url(url)
.post(body)
.addHeader("Content-Type", "application/json")
.addHeader("Accept", "application/x-ndjson")
.addHeader("Authorization", "Bearer {token}")
.build();
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
e.printStackTrace();
}
@Override
public void onResponse(Call call, Response response) throws IOException {
if (!response.isSuccessful()) throw new IOException("Unexpected code " + response);
try (BufferedReader br = new BufferedReader(
new InputStreamReader(response.body().byteStream(), "UTF-8"))) {
String responseLine;
while ((responseLine = br.readLine()) != null) {
System.out.println(responseLine);
}
}
}
});
其他語言可以另外自行改寫,原理都是一樣的。
我們知道,OpenAI 相關的 API 有對應的 system_prompt
的概念,就是給整個模型設置一個預設,比如它叫什么名字等等。本 AI 問答 API 也暴露了這個參數,叫做 preset
,利用它我們可以給模型增加預設,我們用一個例子來體驗下:
這里我們額外添加 preset
字段,內容為 You are a professional artist
,如圖所示:
對應代碼如下:
curl -X POST 'https://api.acedata.cloud/aichat/conversations' \
-H 'accept: application/json' \
-H 'authorization: Bearer {token}' \
-H 'content-type: application/json' \
-d '{
"model": "gpt-3.5",
"stateful": true,
"question": "What can you help me?",
"preset": "You are a professional artist"
}'
運行結果如下:
{
"answer": "As a professional artist, I can offer a range of services and assistance depending on your specific needs. Here are a few ways I can help you:\n\n1. Custom Artwork: If you have a specific vision or idea, I can create custom artwork for you. This can include paintings, drawings, digital art, or any other medium you prefer.\n\n2. Commissioned Pieces: If you have a specific subject or concept in mind, I can create commissioned art pieces tailored to your preferences. This could be for personal enjoyment or as a unique gift for someone special.\n\n3. Art Consultation: If you need guidance on art selection, interior design, or how to showcase and display art in your space, I can provide professional advice to help enhance your aesthetic sense and create a cohesive look."
}
可以看到這里我們告訴 GPT 他是一個機器人,然后問它可以為我們做什么,他就可以扮演一個機器人的角色來回答問題了。
本 AI 也能支持添加附件進行圖片識別,通過 references
傳遞對應圖片鏈接即可,比如我這里有一張蘋果的圖片,如圖所示:
該圖片的鏈接是 https://cdn.acedata.cloud/ht05g0.png,我們直接將其作為 references
參數傳遞即可,同時需要注意的是,模型必須要選擇支持視覺識別的模型,目前支持的是 gpt-4-vision
,所以輸入如下:
對應的代碼如下:
curl -X POST 'https://api.acedata.cloud/aichat/conversations' \
-H 'accept: application/json' \
-H 'authorization: Bearer {token}' \
-H 'content-type: application/json' \
-d '{
"model": "gpt-4-vision",
"question": "How many apples in the picture?",
"references": ["https://cdn.acedata.cloud/ht05g0.png"]
}'
運行結果如下:
{
"answer": "There are 5 apples in the picture."
}
可以看到,我們就成功得到了對應圖片的回答結果。
代碼如下:
curl -X POST 'https://api.acedata.cloud/aichat/conversations' \
-H 'accept: application/json' \
-H 'authorization: Bearer {token}' \
-H 'content-type: application/json' \
-d '{
"model": "gpt-3.5-browsing",
"question": "What's the weather of New York today?"
}'
運行結果如下:
{
"answer": "The weather in New York today is as follows:\n- Current Temperature: 16°C (60°F)\n- High: 16°C (60°F)\n- Low: 10°C (50°F)\n- Humidity: 47%\n- UV Index: 6 of 11\n- Sunrise: 5:42 am\n- Sunset: 8:02 pm\n\nIt's overcast with a chance of occasional showers overnight, and the chance of rain is 50%.\nFor more details, you can visit [The Weather Channel](https://weather.com/weather/tenday/l/96f2f84af9a5f5d452eb0574d4e4d8a840c71b05e22264ebdc0056433a642c84).\n\nIs there anything else you'd like to know?"
}
可以看到,這里它自動聯網搜索了 The Weather Channel 網站,并獲得了里面的信息,然后進一步返回了實時結果。
“
如果對模型回答質量有更高要求,可以將模型更換為
gpt-4-browsing
,回答效果會更好。
文章轉自微信公眾號@進擊的Coder