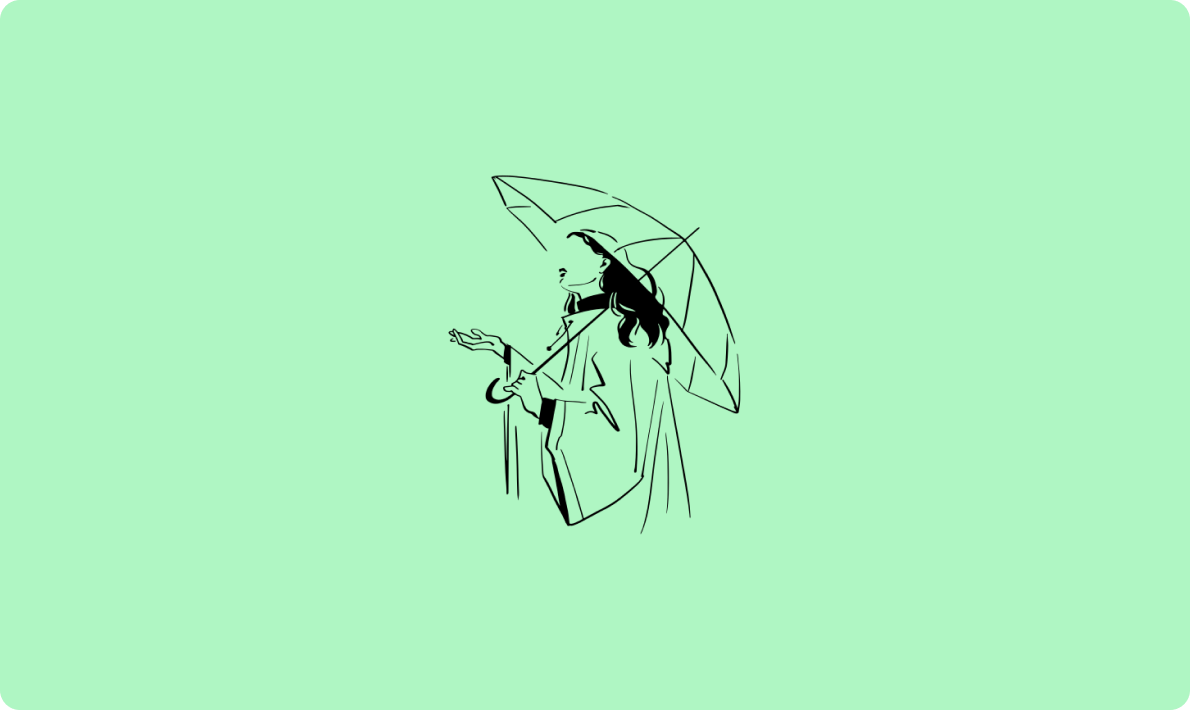
Python調(diào)用墨跡天氣API實(shí)踐指南
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.3.2</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>br.com.erakles</groupId>
<artifactId>spring-openai</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>spring-openai</name>
<description>Demo project to explain the Spring and OpenAI integration</description>
<properties>
<java.version>17</java.version>
<spring-ai.version>1.0.0-M1</spring-ai.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents.client5</groupId>
<artifactId>httpclient5</artifactId>
<version>5.3.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
在您的配置文件(application.properties)中,在屬性中設(shè)置 OpenAI 密鑰openai.api.key
。您還可以在屬性文件中替換模型版本以使用不同的 API 版本,例如gpt-4o-mini
。
spring.application.name=spring-openai
openai.api.url=https://api.openai.com/v1/chat/completions
openai.api.key=YOUR-OPENAI-API-KEY-GOES-HERE
openai.api.model=gpt-3.5-turbo
通過(guò) Java 連接此服務(wù)的一個(gè)棘手部分是,默認(rèn)情況下,它將要求您的 HTTP 客戶端在執(zhí)行此請(qǐng)求時(shí)使用有效證書(shū)。為了解決這個(gè)問(wèn)題,我們將跳過(guò)此驗(yàn)證步驟。
要禁用 JDK 對(duì) HTTPS 請(qǐng)求所需的安全證書(shū)要求,您必須通過(guò)配置類在RestTemplate bean 中包含以下修改:
import org.apache.hc.client5.http.classic.HttpClient;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.client5.http.impl.io.BasicHttpClientConnectionManager;
import org.apache.hc.client5.http.socket.ConnectionSocketFactory;
import org.apache.hc.client5.http.socket.PlainConnectionSocketFactory;
import org.apache.hc.client5.http.ssl.NoopHostnameVerifier;
import org.apache.hc.client5.http.ssl.SSLConnectionSocketFactory;
import org.apache.hc.core5.http.config.Registry;
import org.apache.hc.core5.http.config.RegistryBuilder;
import org.apache.hc.core5.ssl.SSLContexts;
import org.apache.hc.core5.ssl.TrustStrategy;
import org.springframework.boot.web.client.RestTemplateBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.client.HttpComponentsClientHttpRequestFactory;
import org.springframework.web.client.RestTemplate;
import javax.net.ssl.SSLContext;
@Configuration
public class SpringOpenAIConfig {
@Bean
public RestTemplate secureRestTemplate(RestTemplateBuilder builder) throws Exception {
// This configuration allows your application to skip the SSL check
final TrustStrategy acceptingTrustStrategy = (cert, authType) -> true;
final SSLContext sslContext = SSLContexts.custom()
.loadTrustMaterial(null, acceptingTrustStrategy)
.build();
final SSLConnectionSocketFactory sslsf =
new SSLConnectionSocketFactory(sslContext, NoopHostnameVerifier.INSTANCE);
final Registry<ConnectionSocketFactory> socketFactoryRegistry =
RegistryBuilder.<ConnectionSocketFactory>create()
.register("https", sslsf)
.register("http", new PlainConnectionSocketFactory())
.build();
final BasicHttpClientConnectionManager connectionManager =
new BasicHttpClientConnectionManager(socketFactoryRegistry);
HttpClient client = HttpClients.custom()
.setConnectionManager(connectionManager)
.build();
return builder
.requestFactory(() -> new HttpComponentsClientHttpRequestFactory(client))
.build();
}
}
現(xiàn)在我們已經(jīng)準(zhǔn)備好了所有配置,是時(shí)候?qū)崿F(xiàn)一個(gè)處理與 ChatGPT API 通信的服務(wù)了。我正在使用 Spring 組件RestTemplate
,它允許執(zhí)行對(duì) OpenAI 端點(diǎn)的 HTTP 請(qǐng)求。
import org.springframework.beans.factory.annotation.Value;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.MediaType;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
@Service
public class JavaOpenAIService {
@Value("${openai.api.url}")
private String apiUrl;
@Value("${openai.api.key}")
private String apiKey;
@Value("${openai.api.model}")
private String modelVersion;
private final RestTemplate restTemplate;
public JavaOpenAIService(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
/**
* Sends a request to the OpenAI API with the given prompt and returns the response.
*
* @param prompt the question to ask the AI
* @return the response in JSON format
*/
public String ask(String prompt) {
HttpEntity<String> entity = new HttpEntity<>(buildMessageBody(modelVersion, prompt), buildOpenAIHeaders());
return restTemplate
.exchange(apiUrl, HttpMethod.POST, entity, String.class)
.getBody();
}
private HttpHeaders buildOpenAIHeaders() {
HttpHeaders headers = new HttpHeaders();
headers.set("Authorization", "Bearer " + apiKey);
headers.set("Content-Type", MediaType.APPLICATION_JSON_VALUE);
return headers;
}
private String buildMessageBody(String modelVersion, String prompt) {
return String.format("{ \"model\": \"%s\", \"messages\": [{\"role\": \"user\", \"content\": \"%s\"}]}", modelVersion, prompt);
}
}
然后,您可以創(chuàng)建自己的 REST API 來(lái)接收問(wèn)題并將其重定向到您的服務(wù)。
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import br.com.erakles.springopenai.service.SpringOpenService;
@RestController
public class SpringOpenAIController {
private final SpringOpenService springOpenService;
SpringOpenAIController(SpringOpenService springOpenService) {
this.springOpenService = springOpenService;
}
@GetMapping("/chat")
public ResponseEntity<String> sendMessage(@RequestParam String prompt) {
return ResponseEntity.ok(springOpenService.askMeAnything(prompt));
}
}
這些是將您的 Web 應(yīng)用程序與 OpenAI 服務(wù)集成所需的步驟,因此您以后可以通過(guò)添加更多功能(例如向其端點(diǎn)發(fā)送語(yǔ)音、圖像和其他文件)來(lái)改進(jìn)它。
啟動(dòng) Spring Boot 應(yīng)用程序 ( ./mvnw spring-boot:run
) 后,要測(cè)試 Web 服務(wù),您必須運(yùn)行以下 URL http://localhost:8080/ask?promp={add-your-question}。
如果您所有操作正確,您將能夠在響應(yīng)主體中讀取以下結(jié)果:
{
"id": "chatcmpl-9vSFbofMzGkLTQZeYwkseyhzbruXK",
"object": "chat.completion",
"created": 1723480319,
"model": "gpt-3.5-turbo-0125",
"choices": [
{
"index": 0,
"message": {
"role": "assistant",
"content": "Scuba stands for \"self-contained underwater breathing apparatus.\" It is a type of diving equipment that allows divers to breathe underwater while exploring the underwater world. Scuba diving involves using a tank of compressed air or other breathing gas, a regulator to control the flow of air, and various other accessories to facilitate diving, such as fins, masks, and wetsuits. Scuba diving allows divers to explore the underwater environment and observe marine life up close.",
"refusal": null
},
"logprobs": null,
"finish_reason": "stop"
}
],
"usage": {
"prompt_tokens": 12,
"completion_tokens": 90,
"total_tokens": 102
},
"system_fingerprint": null
}
希望本教程能幫助您首次與 OpenAI 互動(dòng),讓您在深入 AI 之旅時(shí)生活更輕松。
原文鏈接:https://dzone.com/articles/integrate-spring-with-open-ai
Python調(diào)用墨跡天氣API實(shí)踐指南
使用 PHP 進(jìn)行 Web 抓取的初學(xué)者指南
一文學(xué)會(huì) API 管理
API 治理:數(shù)字化轉(zhuǎn)型中的控制與合規(guī)
2024年頂級(jí)加密貨幣API:實(shí)時(shí)數(shù)據(jù)和市場(chǎng)分析的終極指南
使用DeepSeek 5步寫出論文提綱
2024年6大最佳 AI 內(nèi)容檢測(cè)器
Python調(diào)用股票API獲取實(shí)時(shí)數(shù)據(jù)
Python 實(shí)時(shí)聊天室搭建:發(fā)布訂閱頻道API實(shí)戰(zhàn)應(yīng)用
對(duì)比大模型API的內(nèi)容創(chuàng)意新穎性、情感共鳴力、商業(yè)轉(zhuǎn)化潛力
一鍵對(duì)比試用API 限時(shí)免費(fèi)