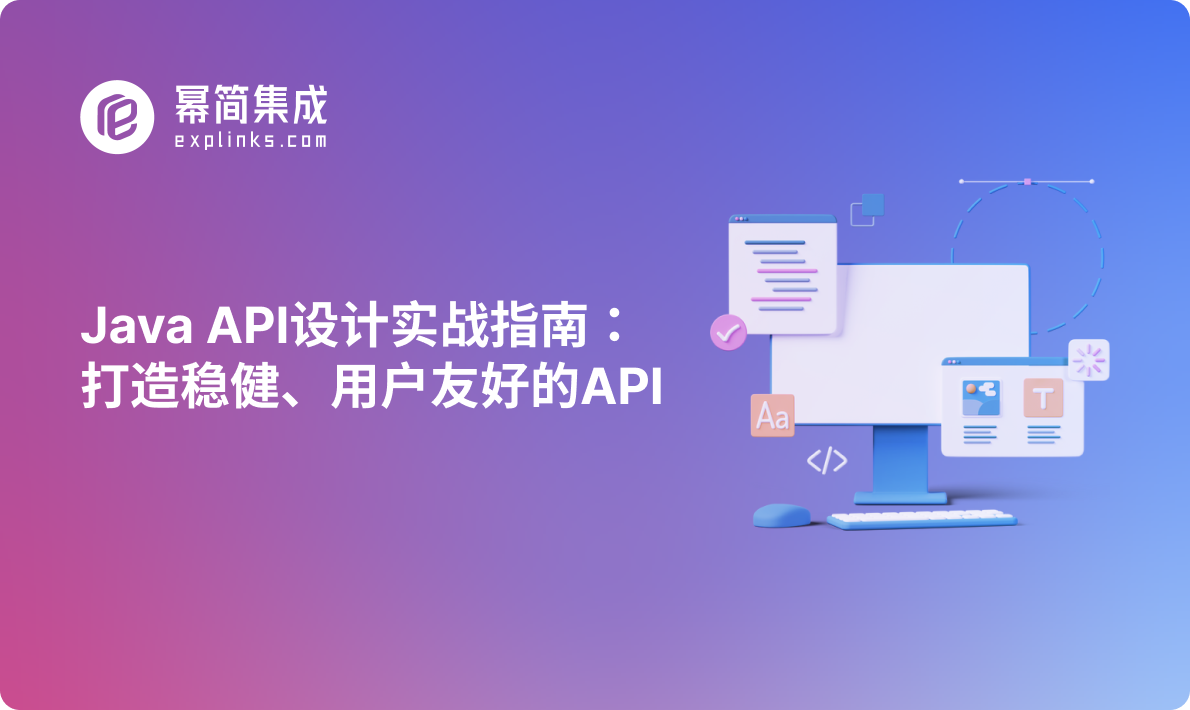
Java?API設計實戰指南:打造穩健、用戶友好的API
!pip show openai | grep Version
Assistant API的Python SDK要求OpenAI版本>1.2.3。
Version: 1.3.7
定義API密鑰。
import json
import os
def show_json(obj):
display(json.loads(obj.model_dump_json()))
os.environ['OPENAI_API_KEY'] = str("Your OpenAI API Key goes here.")
代理已創建完成。
# You can also create Assistants directly through the Assistants API
from openai import OpenAI
client = OpenAI()
assistant = client.beta.assistants.create(
name="History Tutor",
instructions="You are a personal history tutor. Answer questions briefly, in three sentence or less.",
model="gpt-4-1106-preview",
)
show_json(assistant)
在輸出的JSON中。創建代理后,您將看到ID、型號、Assistant命名和其他詳細信息。
{'id': 'asst_qlaTYRSyl9EWeftjKSskdaco',
'created_at': 1702009585,
'description': None,
'file_ids': [],
'instructions': 'You are a personal history tutor. Answer questions briefly, in three sentence or less.',
'metadata': {},
'model': 'gpt-4-1106-preview',
'name': 'History Tutor',
'object': 'assistant',
'tools': []
}
一旦創建了Assistant,就可以通過OpenAI儀表板看到它,并顯示其名稱、說明和ID。
無論您是通過儀表板還是使用API創建Assistant,都需要跟蹤AssistantI D。
首先創建線程。
# Creating a new thread:
thread = client.beta.threads.create()
show_json(thread)
下面是輸出,包括線程ID等。
{'id': 'thread_1flknQB4C8KH4BDYPWsyl0no',
'created_at': 1702009588,
'metadata': {},
'object': 'thread'}
在這里,一條消息被添加到線程中。
# Now we add a message to the thread:
message = client.beta.threads.messages.create(
thread_id=thread.id,
role="user",
content="What year was the USA founded?",
)
show_json(message)
結果如下。
在這里,您需要注意的是,即使每次都沒有發送會話歷史記錄,您仍要為每次運行整個會話歷史記錄支付token費用。
{'id': 'msg_5xOq4FV38cS98ohBpQPbpUiE',
'assistant_id': None,
'content': [{'text': {'annotations': [],
'value': 'What year was the USA founded?'},
'type': 'text'}],
'created_at': 1702009591,
'file_ids': [],
'metadata': {},
'object': 'thread.message',
'role': 'user',
'run_id': None,
'thread_id': 'thread_1flknQB4C8KH4BDYPWsyl0no'}
當前面提到定義run時,必須同時指定Assistant和Thread。
run = client.beta.threads.runs.create(
thread_id=thread.id,
assistant_id=assistant.id,
)
show_json(run)
再次輸出:
{'id': 'run_PnwSECkqDDdjWkQ5P7Hcyfor',
'assistant_id': 'asst_qlaTYRSyl9EWeftjKSskdaco',
'cancelled_at': None,
'completed_at': None,
'created_at': 1702009598,
'expires_at': 1702010198,
'failed_at': None,
'file_ids': [],
'instructions': 'You are a personal history tutor. Answer questions briefly, in three sentence or less.',
'last_error': None,
'metadata': {},
'model': 'gpt-4-1106-preview',
'object': 'thread.run',
'required_action': None,
'started_at': None,
'status': 'queued',
'thread_id': 'thread_1flknQB4C8KH4BDYPWsyl0no',
'tools': []}
與Chat Completions API中的完成不同,創建Run是一個異步操作。它將立即返回運行元數據,其中包括一個 status ,初始設置為 queued 。該值將隨著Assistant執行操作而更新。
下面的循環檢查while循環中的運行狀態,直到運行狀態達到完整狀態。
import time
def wait_on_run(run, thread):
while run.status == "queued" or run.status == "in_progress":
run = client.beta.threads.runs.retrieve(
thread_id=thread.id,
run_id=run.id,
)
time.sleep(0.5)
return run
run = wait_on_run(run, thread)
show_json(run)
低于運行結果。
{'id': 'run_PnwSECkqDDdjWkQ5P7Hcyfor',
'assistant_id': 'asst_qlaTYRSyl9EWeftjKSskdaco',
'cancelled_at': None,
'completed_at': 1702009605,
'created_at': 1702009598,
'expires_at': None,
'failed_at': None,
'file_ids': [],
'instructions': 'You are a personal history tutor. Answer questions briefly, in three sentence or less.',
'last_error': None,
'metadata': {},
'model': 'gpt-4-1106-preview',
'object': 'thread.run',
'required_action': None,
'started_at': 1702009598,
'status': 'completed',
'thread_id': 'thread_1flknQB4C8KH4BDYPWsyl0no',
'tools': []}
一旦運行完成,我們就可以列出線程中的所有消息。
# Now that the Run has completed, list the Messages in the Thread to
# see what got added by the Assistant.
messages = client.beta.threads.messages.list(thread_id=thread.id)
show_json(messages)
再次輸出如下…
{'data': [{'id': 'msg_WhzkHcPnszsmbdrn0H5Ugl7I',
'assistant_id': 'asst_qlaTYRSyl9EWeftjKSskdaco',
'content': [{'text': {'annotations': [],
'value': 'The United States of America was founded in 1776, with the adoption of the Declaration of Independence on July 4th of that year.'},
'type': 'text'}],
'created_at': 1702009604,
'file_ids': [],
'metadata': {},
'object': 'thread.message',
'role': 'assistant',
'run_id': 'run_PnwSECkqDDdjWkQ5P7Hcyfor',
'thread_id': 'thread_1flknQB4C8KH4BDYPWsyl0no'},
{'id': 'msg_5xOq4FV38cS98ohBpQPbpUiE',
'assistant_id': None,
'content': [{'text': {'annotations': [],
'value': 'What year was the USA founded?'},
'type': 'text'}],
'created_at': 1702009591,
'file_ids': [],
'metadata': {},
'object': 'thread.message',
'role': 'user',
'run_id': None,
'thread_id': 'thread_1flknQB4C8KH4BDYPWsyl0no'}],
'object': 'list',
'first_id': 'msg_WhzkHcPnszsmbdrn0H5Ugl7I',
'last_id': 'msg_5xOq4FV38cS98ohBpQPbpUiE',
'has_more': False}
一條消息被附加到線程…
# Create a message to append to our thread
message = client.beta.threads.messages.create(
thread_id=thread.id, role="user", content="Could you give me a little more detail on this?"
)
# Execute our run
run = client.beta.threads.runs.create(
thread_id=thread.id,
assistant_id=assistant.id,
)
# Wait for completion
wait_on_run(run, thread)
# Retrieve all the messages added after our last user message
messages = client.beta.threads.messages.list(
thread_id=thread.id, order="asc", after=message.id
)
show_json(messages)
根據結果,考慮內容價值…
{'data': [{'id': 'msg_oIOfuARjk20zZRn6lAytf0Hz',
'assistant_id': 'asst_qlaTYRSyl9EWeftjKSskdaco',
'content': [{'text': {'annotations': [],
'value': 'Certainly! The founding of the USA is marked by the Declaration of Independence, which was ratified by the Continental Congress on July 4, 1776. This act declared the thirteen American colonies free and independent states, breaking away from British rule.'},
'type': 'text'}],
'created_at': 1702009645,
'file_ids': [],
'metadata': {},
'object': 'thread.message',
'role': 'assistant',
'run_id': 'run_9dWR1QFrN983q1AG1cjcQ9Le',
'thread_id': 'thread_1flknQB4C8KH4BDYPWsyl0no'}],
'object': 'list',
'first_id': 'msg_oIOfuARjk20zZRn6lAytf0Hz',
'last_id': 'msg_oIOfuARjk20zZRn6lAytf0Hz',
'has_more': False}
運行完成后,可以在線程中列出消息。
# Now that the Run has completed, list the Messages in the Thread to see
# what got added by the Assistant.
messages = client.beta.threads.messages.list(thread_id=thread.id)
show_json(messages)
結果再次出現。
{'data': [{'id': 'msg_oIOfuARjk20zZRn6lAytf0Hz',
'assistant_id': 'asst_qlaTYRSyl9EWeftjKSskdaco',
'content': [{'text': {'annotations': [],
'value': 'Certainly! The founding of the USA is marked by the Declaration of Independence, which was ratified by the Continental Congress on July 4, 1776. This act declared the thirteen American colonies free and independent states, breaking away from British rule.'},
'type': 'text'}],
'created_at': 1702009645,
'file_ids': [],
'metadata': {},
'object': 'thread.message',
'role': 'assistant',
'run_id': 'run_9dWR1QFrN983q1AG1cjcQ9Le',
'thread_id': 'thread_1flknQB4C8KH4BDYPWsyl0no'},
{'id': 'msg_dDeGGSj4w3CIVRd5hsQpGHmF',
'assistant_id': None,
'content': [{'text': {'annotations': [],
'value': 'Could you give me a little more detail on this?'},
'type': 'text'}],
'created_at': 1702009643,
'file_ids': [],
'metadata': {},
'object': 'thread.message',
'role': 'user',
'run_id': None,
'thread_id': 'thread_1flknQB4C8KH4BDYPWsyl0no'},
{'id': 'msg_WhzkHcPnszsmbdrn0H5Ugl7I',
'assistant_id': 'asst_qlaTYRSyl9EWeftjKSskdaco',
'content': [{'text': {'annotations': [],
'value': 'The United States of America was founded in 1776, with the adoption of the Declaration of Independence on July 4th of that year.'},
'type': 'text'}],
'created_at': 1702009604,
'file_ids': [],
'metadata': {},
'object': 'thread.message',
'role': 'assistant',
'run_id': 'run_PnwSECkqDDdjWkQ5P7Hcyfor',
'thread_id': 'thread_1flknQB4C8KH4BDYPWsyl0no'},
{'id': 'msg_5xOq4FV38cS98ohBpQPbpUiE',
'assistant_id': None,
'content': [{'text': {'annotations': [],
'value': 'What year was the USA founded?'},
'type': 'text'}],
'created_at': 1702009591,
'file_ids': [],
'metadata': {},
'object': 'thread.message',
'role': 'user',
'run_id': None,
'thread_id': 'thread_1flknQB4C8KH4BDYPWsyl0no'}],
'object': 'list',
'first_id': 'msg_oIOfuARjk20zZRn6lAytf0Hz',
'last_id': 'msg_5xOq4FV38cS98ohBpQPbpUiE',
'has_more': False}