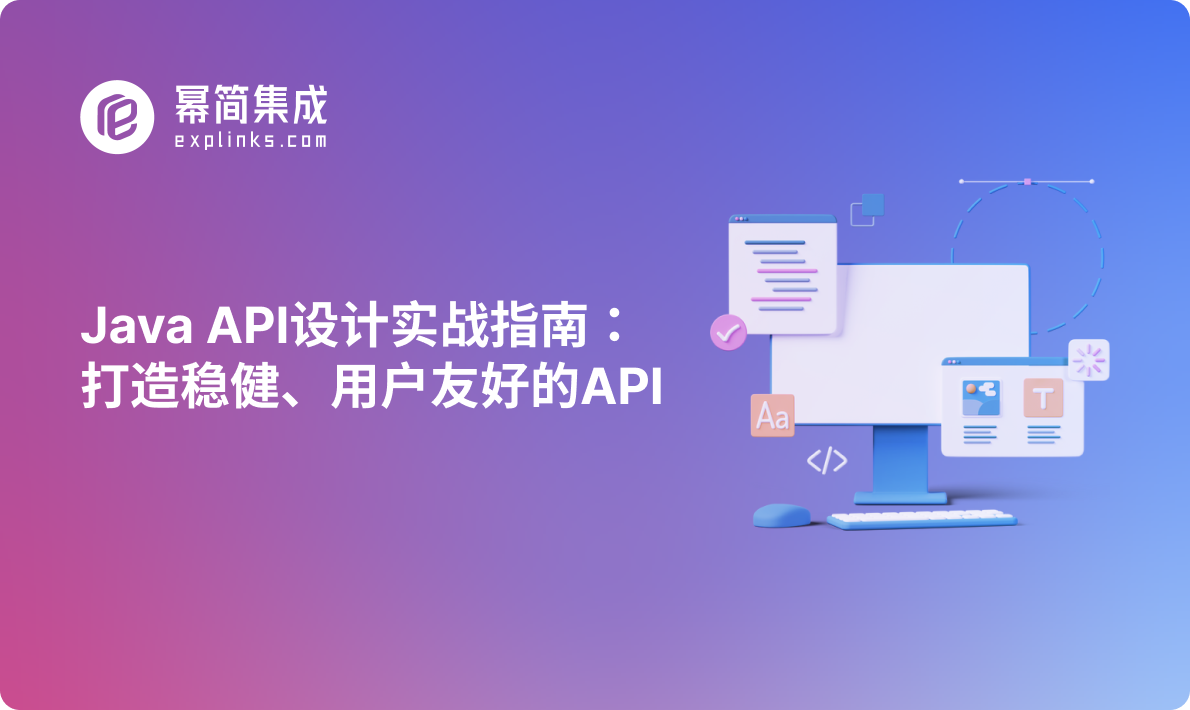
Java?API設計實戰指南:打造穩健、用戶友好的API
[Route("api/[controller]")]
public class MyController : ControllerBase
{
[Authorize] // 需要身份驗證才能訪問此端點
[HttpGet]
public IActionResult Get()
{
// 邏輯在這里
return Ok("經過身份驗證的用戶可以訪問此資源.");
}
}
基于令牌的身份驗證是一種被廣泛使用的方法,通過向已認證的用戶頒發唯一令牌,隨后?API?請求憑此令牌進行驗證。最常用的令牌生成機制是?JWT?令牌(JSON?Web?Token)。以下是使用?C#?創建?JWT?令牌以對用戶進行身份驗證的示例。
public IActionResult Authenticate()
{
var tokenHandler = new JwtSecurityTokenHandler();
var key = Encoding.ASCII.GetBytes("your_secret_key");
var tokenDescriptor = new SecurityTokenDescriptor
{
Subject = new ClaimsIdentity(new Claim[]
{
new Claim(ClaimTypes.Name, "Shubhadeep Chattopadhyay"),
// 根據需要添加更多
}),
Expires = DateTime.UtcNow.AddDays(1),
SigningCredentials = new SigningCredentials(new SymmetricSecurityKey(key), SecurityAlgorithms.HmacSha256Signature)
};
var token = tokenHandler.CreateToken(tokenDescriptor);
var tokenString = tokenHandler.WriteToken(token);
return Ok(new { Token = tokenString });
}
API?密鑰是授予用戶或應用程序以訪問特定?API?的唯一標識符。它們充當一種簡單的身份驗證形式,需要在?API?調用時作為?HTTP?標頭信息傳遞。以下是使用?C#?驗證密鑰的示例。在實際實現時,邏輯應該是集中的。
[ApiController]
[Route("api/[controller]")]
public class MyController : ControllerBase
{
private const string ApiKey = "your_api_key";
[HttpGet]
public IActionResult Get()
{
var apiKey = Request.Headers["Api-Key"].FirstOrDefault();
if (apiKey != ApiKey)
return Unauthorized();
// 這里的邏輯
return Ok("在Startup.cs中,將以下內容添加到Configure方法中.");
}
}
速率限制,是對用戶或應用程序在特定時間范圍內可以向 API 發出請求數量的限制。這有助于防止濫用行為,確保資源被公平合理地利用。
下面是使用?ASP.Net?Core?中間件實現速率限制的示例,每分鐘僅允許?100?個調用。
public class RateLimitingMiddleware
{
private readonly RequestDelegate _next;
private readonly int _requestLimit;
private readonly TimeSpan _timeFrame;
private readonly ConcurrentDictionary<string, int> _requestCount = new ConcurrentDictionary<string, int>();
public RateLimitingMiddleware(RequestDelegate next, int requestLimit, TimeSpan timeFrame)
{
_next = next;
_requestLimit = requestLimit;
_timeFrame = timeFrame;
}
public async Task InvokeAsync(HttpContext context)
{
var ipAddress = context.Connection.RemoteIpAddress.ToString();
var currentTime = DateTime.UtcNow;
if (_requestCount.TryGetValue(ipAddress, out var count) && (currentTime - TimeSpan.FromMinutes(1)) < _timeFrame)
{
if (count > _requestLimit)
{
context.Response.StatusCode = (int)HttpStatusCode.TooManyRequests;
return;
}
_requestCount[ipAddress] = ++count;
}
else
{
_requestCount[ipAddress] = 1;
}
await _next(context);
}
}
// In Startup.cs, add the following to the Configure method:
app.UseMiddleware<RateLimitingMiddleware>(requestLimit: 100, timeFrame: TimeSpan.FromMinutes(1));
正確的輸入驗證對于防止注入攻擊和數據操縱至關重要。始終驗證和清理傳入數據,以確保數據的完整性和安全性。
以下是使用?ASP.NET?Core?數據注釋進行輸入驗證的示例。如果請求正文無效,則不會接受并返回錯誤請求。
public class UserController : ControllerBase
{
[HttpPost]
public IActionResult CreateUser([FromBody] User user)
{
if (!ModelState.IsValid)
return BadRequest(ModelState);
// Your logic to create the user
return Ok("User created successfully.");
}
}
public class User
{
[Required]
public string Username { get; set; }
[Required]
[EmailAddress]
public string Email { get; set; }
[Required]
[StringLength(8, MinimumLength = 4)]
public string Password { get; set; }
}
傳輸層安全性 (TLS) 或安全套接字層 (SSL) 加密可確保客戶端和 API 服務器之間的安全通信。
以下是在?ASP.NET?Core?啟動類中啟用?HTTPS?的示例。
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
// Other service configurations
services.AddHttpsRedirection(options =>
{
options.HttpsPort = 443; // Default HTTPS port
});
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// Other app configurations
app.UseHttpsRedirection();
}
}
CORS 防止未經授權的域外訪問您的 API。對于所有開發人員來說,這是非常常見的做法,僅允許特定域請求才能被處理。
以下是在?ASP.NET?中配置?CORS?的示例。
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
// Other service configurations
services.AddCors(options =>
{
options.AddPolicy("AllowMyDomain", builder =>
{
builder.WithOrigins("https://www.knowndoamin.com")
.AllowAnyMethod()
.AllowAnyHeader();
});
});
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// Other app configurations
app.UseCors("AllowMyDomain");
}
}
全面的日志記錄和監控有助于深入了解 API 的使用情況,發現潛在的安全漏洞和性能問題。
以下是使用?ASP.Net?和?Serilog?啟用日志記錄的示例。
// In Startup.cs
public class Startup
{
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// Other app configurations
Log.Logger = new LoggerConfiguration()
.WriteTo.File("log.txt")
.CreateLogger();
app.UseSerilogRequestLogging();
// More middleware and configurations
}
}
除了上述流程,API?安全最佳實踐還應關注以下四點:
● 定期更新和修補依賴項和庫。
● 采用最小權限原則,僅授予必要的權限。
● 使用安全密碼散列算法(例如 bcrypt)來存儲密碼。
● 對關鍵操作實施雙因素身份驗證。
在研發流程之外,開發者也可以采用API集成平臺更好地關注API安全。比如,API集成平臺可以幫助設置訪問控制策略,并提供監控和日志記錄功能,實時預警,幫助開發者監控API使用情況并及時發現異常行為。
盡管確保?API?安全是一項多方面的任務,但保護敏感數據并維護用戶和客戶的信任至關重要。本文探討了?C#?中的各種?API?安全機制,包括身份驗證、基于令牌的身份驗證、API?密鑰、速率限制、輸入驗證、TLS/SSL?加密、CORS、日志記錄和監控。通過整合這些最佳實踐,開發人員可以構建強大且安全的?API,從而為更安全的數字生態系統做出貢獻。
原文鏈接:Best Practices of API Security