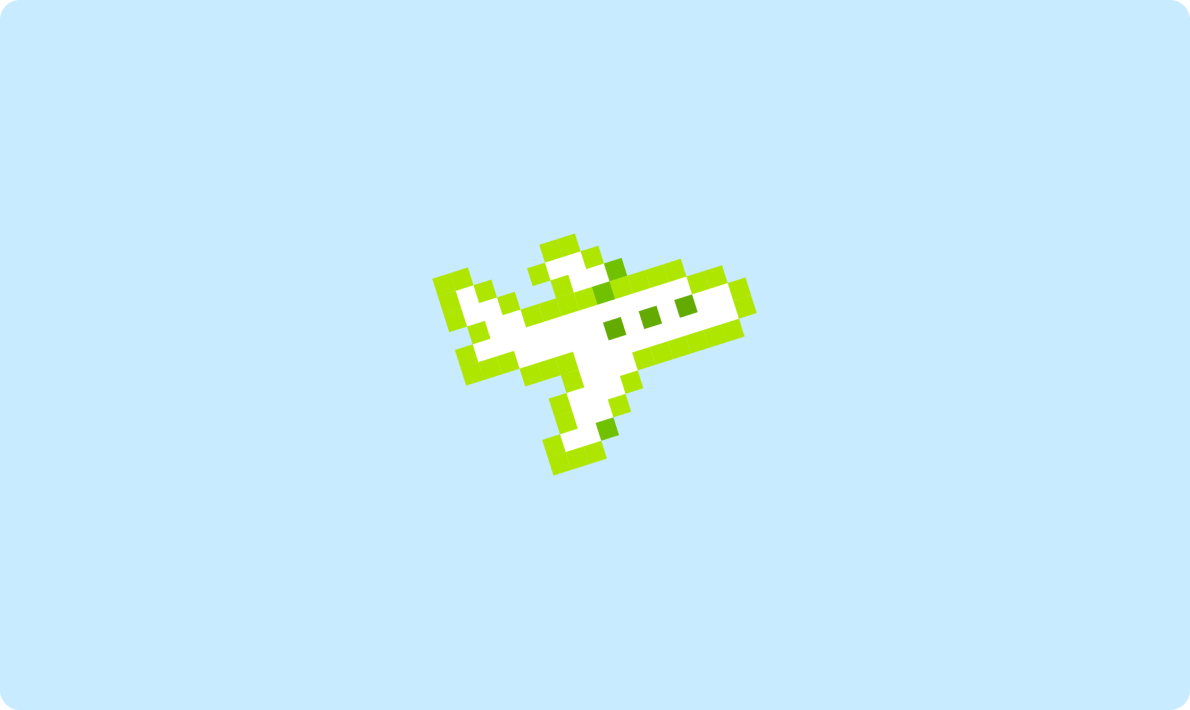
實時航班追蹤背后的技術:在線飛機追蹤器的工作原理
接口的實現可以選擇多種技術棧,例如:
實操建議:
接口通常需要與數據庫進行交互。以下是常見的數據庫類型:
實操示例(使用 Express.js 和 MongoDB):
const express = require('express');
const mongoose = require('mongoose');
const app = express();
const port = 3000;
// 連接 MongoDB
mongoose.connect('mongodb://localhost:27017/mydb', { useNewUrlParser: true, useUnifiedTopology: true });
// 定義用戶模型
const UserSchema = new mongoose.Schema({
name: String,
email: String,
age: Number
});
const User = mongoose.model('User', UserSchema);
// 獲取所有用戶
app.get('/users', async (req, res) => {
const users = await User.find();
res.json(users);
});
// 創建用戶
app.post('/users', async (req, res) => {
const user = new User(req.body);
await user.save();
res.status(201).json(user);
});
app.listen(port, () => {
console.log(Server is running on http://localhost:${port}
);
});
良好的錯誤處理機制可以提高接口的健壯性。以下是一些常見的錯誤處理策略:
{ "error": "Not Found", "message": "User not found" }
。實操示例:
app.use((err, req, res, next) => {
res.status(500).json({ error: 'Internal Server Error', message: err.message });
});
單元測試是針對接口的最小可測試單元(如單個函數或方法)進行的測試。常用的測試框架包括 Mocha、Jest、PyTest 等。
實操示例(使用 Jest):
const { add } = require('./math');
test('adds 1 + 2 to equal 3', () => {
expect(add(1, 2)).toBe(3);
});
集成測試是測試多個模塊或服務之間的交互。可以使用 Postman、SoapUI 等工具進行接口的集成測試。
實操示例(使用 Postman):
http://localhost:3000/users
。pm.expect(pm.response.code).to.equal(200);
。自動化測試可以提高測試效率,減少人為錯誤。可以使用 Jenkins、Travis CI 等工具進行持續集成和持續部署(CI/CD)。
實操示例(使用 Travis CI):
language: node_js
node_js:
- "14"
script:
- npm test
緩存是提高接口性能的有效手段。可以使用 Redis、Memcached 等內存數據庫緩存頻繁訪問的數據。
實操示例(使用 Redis):
const redis = require('redis');
const client = redis.createClient();
app.get('/users/:id', async (req, res) => {
const { id } = req.params;
const cacheKey = user:${id}
;
client.get(cacheKey, async (err, data) => {
if (data) {
res.json(JSON.parse(data));
} else {
const user = await User.findById(id);
client.setex(cacheKey, 3600, JSON.stringify(user));
res.json(user);
}
});
});
負載均衡可以將請求分發到多個服務器,提高系統的吞吐量和可用性。常用的負載均衡器包括 Nginx、HAProxy 等。
實操示例(使用 Nginx):
http {
upstream myapp {
server 127.0.0.1:3000;
server 127.0.0.1:3001;
}
server {
listen 80;
location / {
proxy_pass http://myapp;
}
}
}
自動生成接口文檔可以提高文檔的準確性和維護性。常用的工具包括 Swagger、APIBlueprint 等。
實操示例(使用 Swagger):
const swaggerJsDoc = require('swagger-jsdoc');
const swaggerUi = require('swagger-ui-express');
const swaggerOptions = {
swaggerDefinition: {
info: {
title: 'User API',
version: '1.0.0',
description: 'User API Information'
},
basePath: '/'
},
apis: ['./routes/*.js']
};
const swaggerDocs = swaggerJsDoc(swaggerOptions);
app.use('/api-docs', swaggerUi.serve, swaggerUi.setup(swaggerDocs));
接口開發是一個系統化的工程,涉及設計、實現、測試、優化和文檔等多個環節。通過本文的指南和思維導圖,開發者可以全面掌握接口開發的流程和技巧,構建高效、可靠的系統。希望本文能為您的接口開發實踐提供有價值的參考,助力您打造卓越的產品。