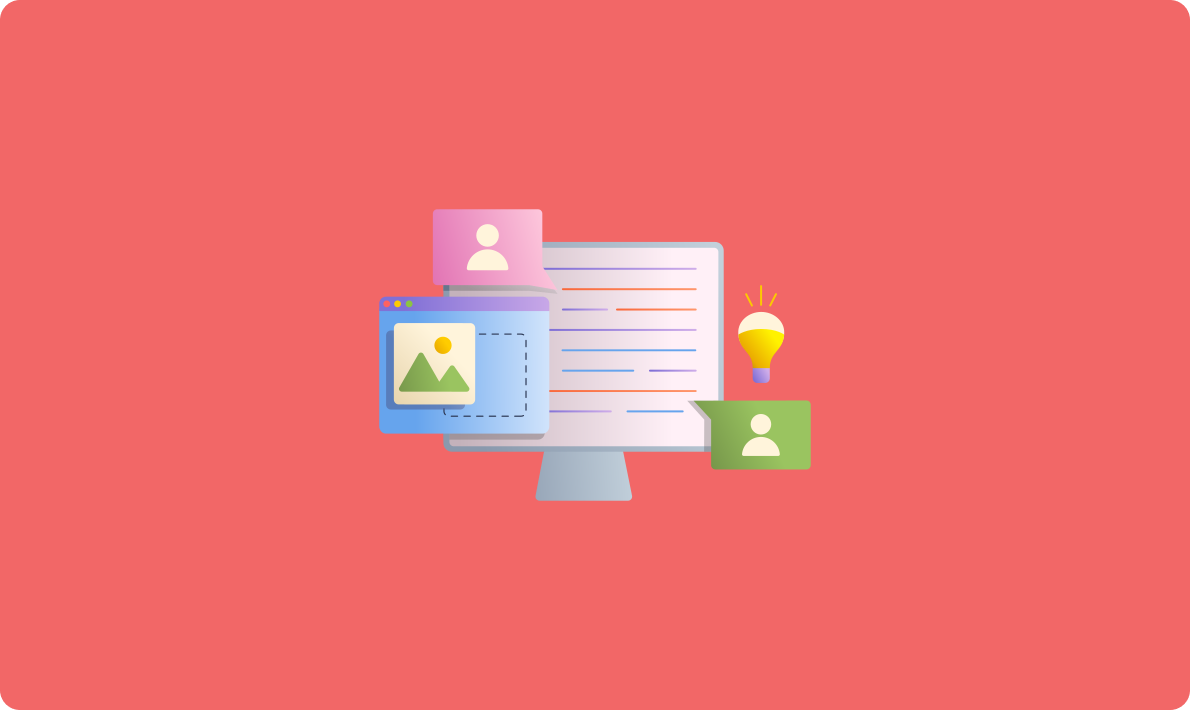
使用API安全的基本工具和最佳實踐預防API攻擊
2import base64
3import json
4
5# 獲取Access Token
6def get_access_token(api_key, secret_key):
7 url = 'https://aip.baidubce.com/oauth/2.0/token'
8 params = {
9 'grant_type': 'client_credentials',
10 'client_id': api_key,
11 'client_secret': secret_key
12 }
13 response = requests.post(url, data=params)
14 return response.json()['access_token']
15
16# 調用實時語音識別服務
17def call_asr(access_token, audio_file_path):
18 url = 'https://vop.baidu.com/server_api'
19 params = {
20 'lan': 'zh',
21 'token': access_token,
22 'cuid': 'your_unique_device_id',
23 'dev_pid': '1536'
24 }
25
26 # 讀取音頻文件
27 with open(audio_file_path, 'rb') as f:
28 audio_data = f.read()
29
30 # 編碼音頻數據
31 audio_base64 = base64.b64encode(audio_data).decode()
32
33 # 發送POST請求
34 headers = {'Content-Type': 'application/json'}
35 data = {
36 'format': 'wav',
37 'rate': 16000,
38 'channel': 1,
39 'speech': audio_base64,
40 'len': len(audio_data),
41 'dev_pid': 1536
42 }
43
44 response = requests.post(url, data=json.dumps(data), headers=headers)
45 return response.json()
46
47# 使用示例
48api_key = 'your_api_key'
49secret_key = 'your_secret_key'
50audio_file_path = 'path_to_your_audio_file.wav'
51
52access_token = get_access_token(api_key, secret_key)
53result = call_asr(access_token, audio_file_path)
54print(result)
php
深色版本
1<?php
2// 獲取Access Token
3function get_access_token($api_key, $secret_key) {
4 $url = 'https://aip.baidubce.com/oauth/2.0/token';
5 $params = [
6 'grant_type' => 'client_credentials',
7 'client_id' => $api_key,
8 'client_secret' => $secret_key
9 ];
10 $ch = curl_init();
11 curl_setopt($ch, CURLOPT_URL, $url);
12 curl_setopt($ch, CURLOPT_POST, true);
13 curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($params));
14 curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
15 $response = curl_exec($ch);
16 curl_close($ch);
17 return json_decode($response, true)['access_token'];
18}
19
20// 調用實時語音識別服務
21function call_asr($access_token, $audio_file_path) {
22 $url = 'https://vop.baidu.com/server_api';
23 $params = [
24 'lan' => 'zh',
25 'token' => $access_token,
26 'cuid' => 'your_unique_device_id',
27 'dev_pid' => '1536'
28 ];
29
30 // 讀取音頻文件
31 $audio_data = file_get_contents($audio_file_path);
32
33 // 編碼音頻數據
34 $audio_base64 = base64_encode($audio_data);
35
36 // 發送POST請求
37 $headers = ['Content-Type: application/json'];
38 $data = [
39 'format' => 'wav',
40 'rate' => 16000,
41 'channel' => 1,
42 'speech' => $audio_base64,
43 'len' => strlen($audio_data),
44 'dev_pid' => 1536
45 ];
46
47 $ch = curl_init();
48 curl_setopt($ch, CURLOPT_URL, $url);
49 curl_setopt($ch, CURLOPT_POST, true);
50 curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
51 curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
52 curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
53 $response = curl_exec($ch);
54 curl_close($ch);
55 return json_decode($response, true);
56}
57
58// 使用示例
59$api_key = 'your_api_key';
60$secret_key = 'your_secret_key';
61$audio_file_path = 'path_to_your_audio_file.wav';
62
63$access_token = get_access_token($api_key, $secret_key);
64$result = call_asr($access_token, $audio_file_path);
65var_dump($result);
66?>
冪簡集成是國內領先的API集成管理平臺,專注于為開發者提供全面、高效、易用的API集成解決方案。冪簡API平臺可以通過以下兩種方式找到所需API:通過關鍵詞搜索API(例如,輸入’AI語音‘這類品類詞,更容易找到結果)、或者從API Hub分類頁進入尋找。
此外,冪簡集成博客會編寫API入門指南、多語言API對接指南、API測評等維度的文章,讓開發者快速使用目標API。