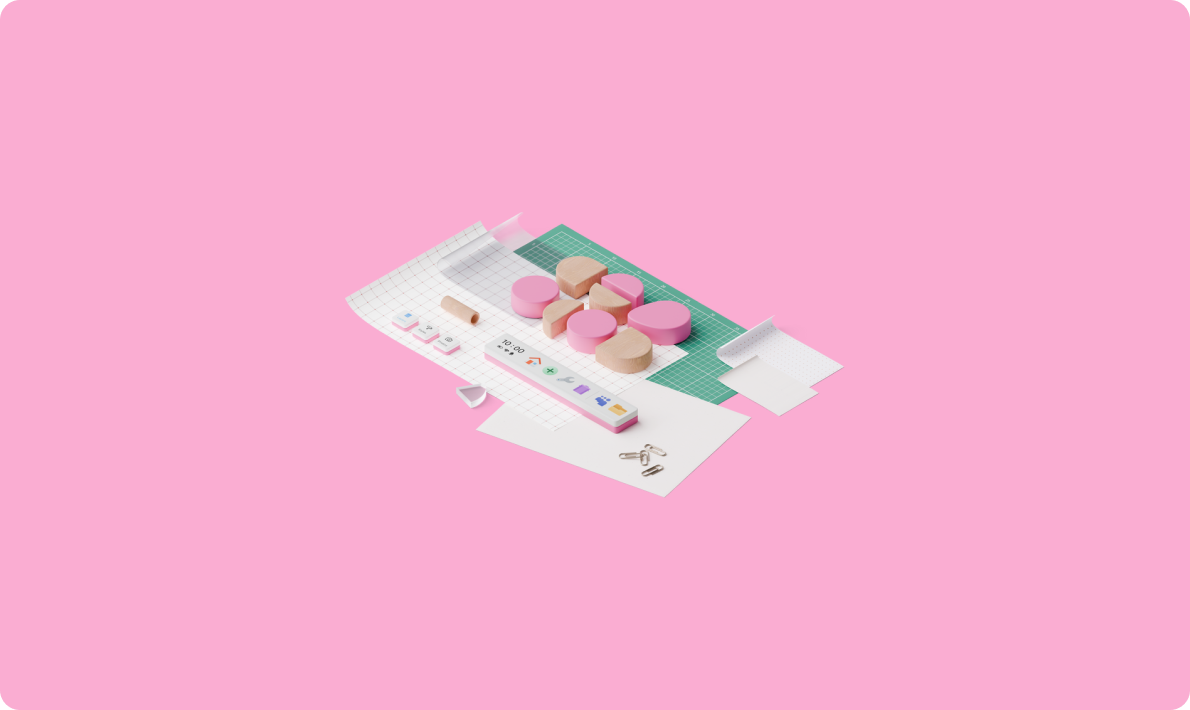
Web應用程序和API安全的新規則
import java.net.HttpURLConnection;
import java.net.URL;
public class ApiExample {
public static void main(String[] args) {
try {
URL url = new URL("https://open.explinks.com/v2/scd2024041173471c54e623/multimodal-large-language-model");
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("POST");
// 設置請求頭信息
con.setRequestProperty("Content-Type", "application/json");
con.setRequestProperty("X-Mce-Signature", "AppCode/your_actual_app_code_here");
// 發送POST請求必須設置
con.setDoOutput(true);
// 獲取輸出流并寫入請求體
try(OutputStream os = con.getOutputStream()) {
String jsonInputString = "{\"key1\":\"value1\"}";
byte[] input = jsonInputString.getBytes("utf-8");
os.write(input, 0, input.length);
}
// 讀取響應
try(BufferedReader br = new BufferedReader(
new InputStreamReader(con.getInputStream(), "utf-8"))) {
StringBuilder response = new StringBuilder();
String responseLine = null;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
System.out.println(response.toString());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
以下是在JavaScript中調用360多模態大語言模型API的示例:
const axios = require('axios');
const postData = {
key1: 'value1'
};
axios.post('https://open.explinks.com/v2/scd2024041173471c54e623/multimodal-large-language-model', postData, {
headers: {
'Content-Type': 'application/json',
'X-Mce-Signature': 'AppCode/your_actual_app_code_here'
}
}).then(response => {
console.log(response.data);
}).catch(error => {
console.error('Error during API call:', error);
});
如果您在考慮替換或補充360多模態大語言模型API時,以下是一些流行的AI平臺和庫,它們提供了類似的服務和功能:
對于紫東太初大模型,以下是一個集成示例,展示如何在Java中調用API進行多模態任務處理:
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Base64;
public class ZiDongTaiChuApiExample {
public static void main(String[] args) {
try {
String apiEndpoint = "http://www.dlbhg.com/api/scd202406267270240e7202"; // 紫東太初大模型API端點
// 替換以下內容為你的請求數據和認證信息
String requestData = "<Your Request Data Here>";
String authToken = "Bearer <Your Auth Token Here>";
// 創建URL對象
URL url = new URL(apiEndpoint);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 設置請求方法和請求頭
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("Authorization", authToken);
connection.setDoOutput(true);
// 獲取輸出流并發送請求體
try(OutputStream os = connection.getOutputStream()) {
byte[] input = requestData.getBytes("utf-8");
os.write(input, 0, input.length);
}
// 讀取響應
try(BufferedReader br = new BufferedReader(new InputStreamReader(connection.getInputStream(), "utf-8"))) {
StringBuilder response = new StringBuilder();
String responseLine = null;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
System.out.println(response.toString());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
請注意,上述代碼僅為示例,實際集成時需要替換requestData
和authToken
等占位符為實際的值。此外,紫東太初大模型的具體API細節(如端點URL、請求參數、認證方式等)可能有所不同,需要根據紫東太初大模型的官方文檔進行調整。通過這些示例,你可以根據自己的需求選擇合適的平臺和庫來集成AI服務。
冪簡集成是國內領先的API集成管理平臺,專注于為開發者提供全面、高效、易用的API集成解決方案。冪簡API平臺可以通過以下兩種方式找到所需API:通過關鍵詞搜索API(例如,輸入’多模態大模型‘這類品類詞,更容易找到結果)、或者從API Hub分類頁進入尋找。
此外,冪簡集成博客會編寫API入門指南、多語言API對接指南、API測評等維度的文章,讓開發者快速使用目標API。